sofia_redux.pipeline: Data Reduction Pipelines for SOFIA¶
The sofia_redux.pipeline
package is a data reduction interface for
astronomy pipelines. It provides interactive and command-line interfaces to
astronomical algorithms.
This package was developed to support data reduction pipelines for the SOFIA telescope, but is designed to be a general-purpose interface package to any arbitrary series of data reduction steps.
Getting Started¶
Running SOFIA pipelines¶
Out of the box, Redux provides two command-line scripts:
redux (
sofia_redux.pipeline.sofia.redux_app
): An interactive graphical interface (GUI) for the SOFIA pipelines.redux_pipe (
sofia_redux.pipeline.sofia.redux_pipe
): A command-line interface to the SOFIA pipelines.
In general, Redux works by reading in input data, deciding which reduction to run, then running a pre-defined set of reduction steps.
The GUI allows interactive parameter editing, and intermediate product display for each step.
To begin a reduction, start the GUI by typing redux
, then load in a set of data with
the File->Open New Reduction menu.
The command-line interface allows fully automatic pipeline reductions. To begin a reduction
with the automatic pipeline, type redux_pipe
and provide the file names of the data
to reduce on the command line. Non-default parameters can also be provided by specifying
a configuration file in INI format.
Displaying FITS data¶
This package also provides a stand-alone front end to some of its display tools
(sofia_redux.pipeline.gui.qad
). The command line script qad (sofia_redux.pipeline.gui.qad.qad_app
) starts a
small GUI that allows interactive display of FITS images and spectra in DS9 and
a native spectral viewer (sofia_redux.visualization.eye
). To use it, DS9
must be installed (see http://ds9.si.edu/), and the ds9
executable
must be available in the PATH environment variable.
To use the QAD, start up the GUI by typing qad
at the command line,
then double-click on a FITS image to display it. Display preferences can be
set from the ‘Settings’ menu
Developing new pipelines¶
Redux is designed to be a development platform, for running any sequence of data reduction steps. To use Redux to develop a new pipeline, the following are necessary:
A reduction class that inherits from the
sofia_redux.pipeline.Reduction
class. This class defines all data reduction steps, and the order in which they should run.A parameter class that inherits from the
sofia_redux.pipeline.Parameters
class. This class should provide default parameters for the steps defined in the reduction object.A reduction object chooser class that inherits from the
sofia_redux.pipeline.Chooser
class. The chooser decides from input data which reduction object to instantiate.A configuration class that inherits from
sofia_redux.pipeline.Configuration
. This class provides default parameters for the interface, such as default log file names, and specifies the reduction chooser.A pipe script that instantiates a
sofia_redux.pipeline.Pipe
object with the appropriate configuration.An application script that instantiates a
sofia_redux.pipeline.Application
object with the appropriate configuration.
Optionally, custom viewers may also be defined for displaying intermediate data
products. These should inherit from the sofia_redux.pipeline.Viewer
class.
See the sofia_redux.pipeline.sofia
module for examples of all these classes and scripts.
Architecture¶
Redux is designed to be a light-weight interface to data reduction pipelines. It contains the definitions of how reduction algorithms should be called for any given instrument, mode, or pipeline, in either a command-line interface (CLI) or graphical user interface (GUI) mode, but it does not contain the reduction algorithms themselves.
Redux is organized around the principle that
any data reduction procedure can be accomplished by running a linear
sequence of data reduction steps. It relies on a Reduction
class that
defines what these steps are and in which order they should be run
(the reduction “recipe”). Reductions have an associated Parameter
class that defines what parameters the steps may accept. Because
reduction classes share common interaction methods, they can be
instantiated and called from a completely generic front-end GUI,
which provides the capability to load in raw data files, and then:
set the parameters for a reduction step,
run the step on all input data,
display the results of the processing,
and repeat this process for every step in sequence to complete the
reduction on the loaded data. In order to choose the correct
reduction object for a given data set, the interface uses a Chooser
class, which reads header information from loaded input files and
uses it to decide which reduction object to instantiate and return.
The GUI is a PyQt application, based around the Application
class. Because the GUI operations are completely separate from the
reduction operations, the automatic pipeline script is simply a wrapper
around a reduction object: the Pipe
class uses the Chooser to
instantiate the Reduction, then calls its reduce method, which calls
each reduction step in order and reports any output files generated.
Both the Application and Pipe classes inherit from a common Interface
class that holds reduction objects and defines the methods for
interacting with them. The Application
class additionally may
start and update custom data viewers associated with the
data reduction; these should inherit from the Redux Viewer
class.
All reduction classes inherit from the generic Reduction
class,
which defines the common interface for all reductions: how parameters
are initialized and modified, how each step is called.
Each specific reduction class must then define
each data reduction step as a method that calls the appropriate
algorithm.
The reduction methods may contain any code necessary to accomplish the data reduction step. Typically, a reduction method will contain code to fetch the parameters for the method from the object’s associated Parameters class, then will call an external data reduction algorithm with appropriate parameter values, and store the results in the ‘input’ attribute to be available for the next processing step. If processing results in data that can be displayed, it should be placed in the ‘display_data’ attribute, in a format that can be recognized by the associated Viewers. The Redux GUI checks this attribute at the end of each data reduction step and displays the contents via the Viewer’s ‘display’ method.
Parameters for data reduction are stored as a list of ParameterSet
objects, one for each reduction step. Parameter sets contain the key,
value, data type, and widget type information for every parameter.
A Parameters
class may generate these parameter sets by
defining a default dictionary that associates step names with parameter
lists that define these values. This dictionary may be defined directly
in the Parameters class, or may be read in from an external configuration
file or software package, as appropriate for the reduction.
Usage¶
Automatic Mode Execution¶
The DPS pipeline infrastructure runs a pipeline on previously-defined reduction groups as a fully-automatic black box. To do so, it creates an input manifest (infiles.txt) that contains relative paths to the input files (one per line). The command-line interface to the pipeline is run as:
redux_pipe infiles.txt
The command-line interface will read in the specified input files, use their headers to determine the observation mode, and accordingly the steps to run and any intermediate files to save. Output files are written to the current directory, from which the pipeline was called. After reduction is complete, the script will generate an output manifest (outfiles.txt) containing the relative paths to all output FITS files generated by the pipeline.
Optionally, in place of a manifest file, file paths to input files may be directly specified on the command line. Input files may be raw FITS files, or may be intermediate products previously produced by the pipeline. For example, this command will complete the reduction for a set of FITS files in the current directory, previously reduced through the calibration step of the pipeline:
redux_pipe *CAL*.fits
To customize batch reductions from the command line, the redux_pipe interface accepts a configuration file on the command line. This file may contain any subset of the full configuration file, specifying any non-default parameters for pipeline steps. An output directory for pipeline products and the terminal log level may also be set on the command line.
The full set of optional command-line parameters accepted by the redux_pipe interface are:
-h, --help show this help message and exit
-c CONFIG, --configuration CONFIG
Path to Redux configuration file.
-o OUTDIR, --out OUTDIR
Path to output directory.
-l LOGLEVEL, --loglevel LOGLEVEL
Log level.
Manual Mode Execution¶
In manual mode, the pipeline may be run interactively, via a graphical user interface (GUI) provided by the Redux package. The GUI is launched by the command:
redux
entered at the terminal prompt (Fig. 124). The GUI allows output directory specification, but it may write initial or temporary files to the current directory, so it is recommended to start the interface from a location to which the user has write privileges.
From the command line, the redux interface accepts an optional config file (-c) or log level specification (-l), in the same way the redux_pipe command does. Any pipeline parameters provided to the interface in a configuration file will be used to set default values; they will still be editable from the GUI.
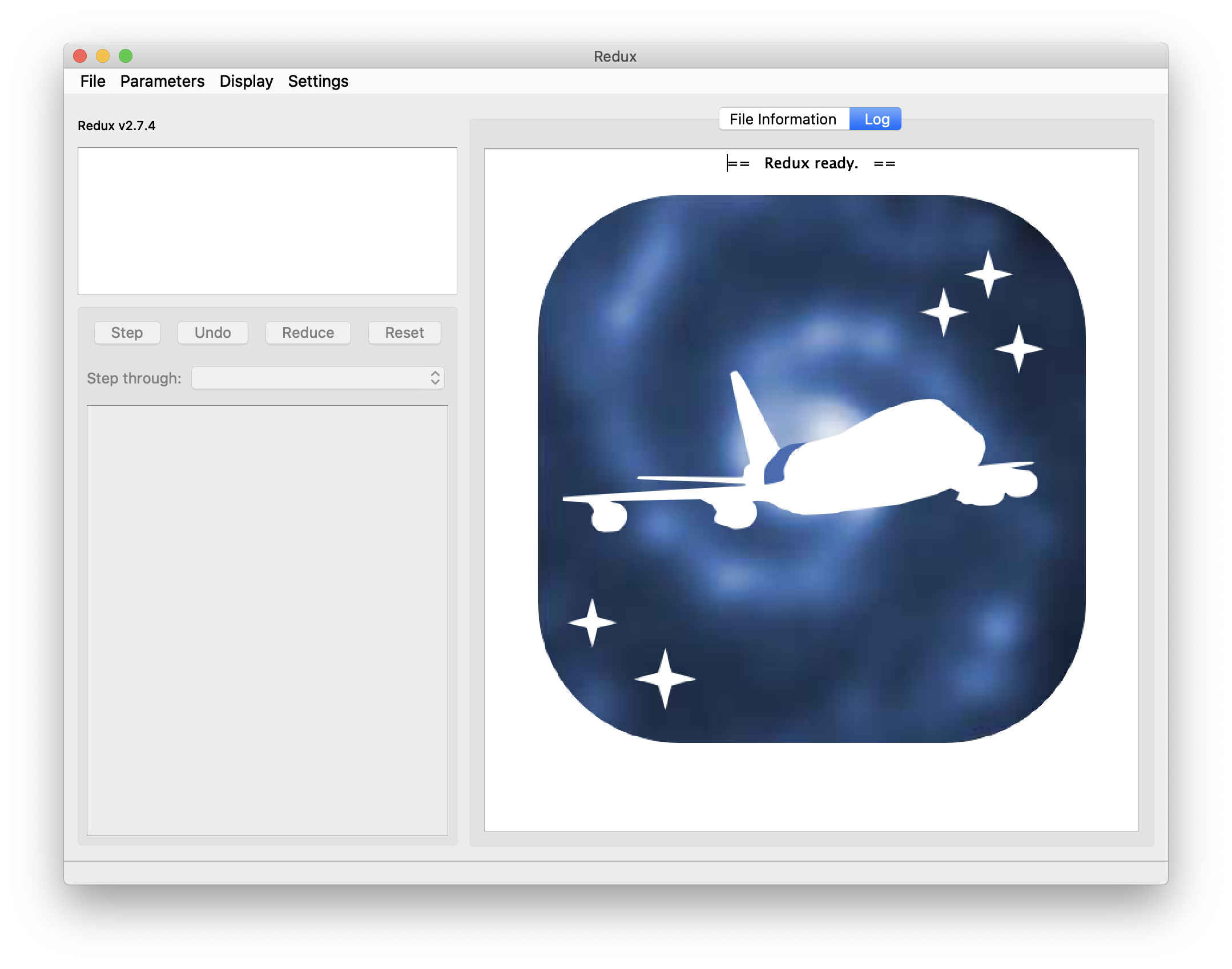
Fig. 124 Redux GUI startup.¶
Basic Workflow¶
To start an interactive reduction, select a set of input files, using the File menu (File->Open New Reduction). This will bring up a file dialog window (see Fig. 125). All files selected will be reduced together as a single reduction set.
Redux will decide the appropriate reduction steps from the input files, and load them into the GUI, as in Fig. 126.
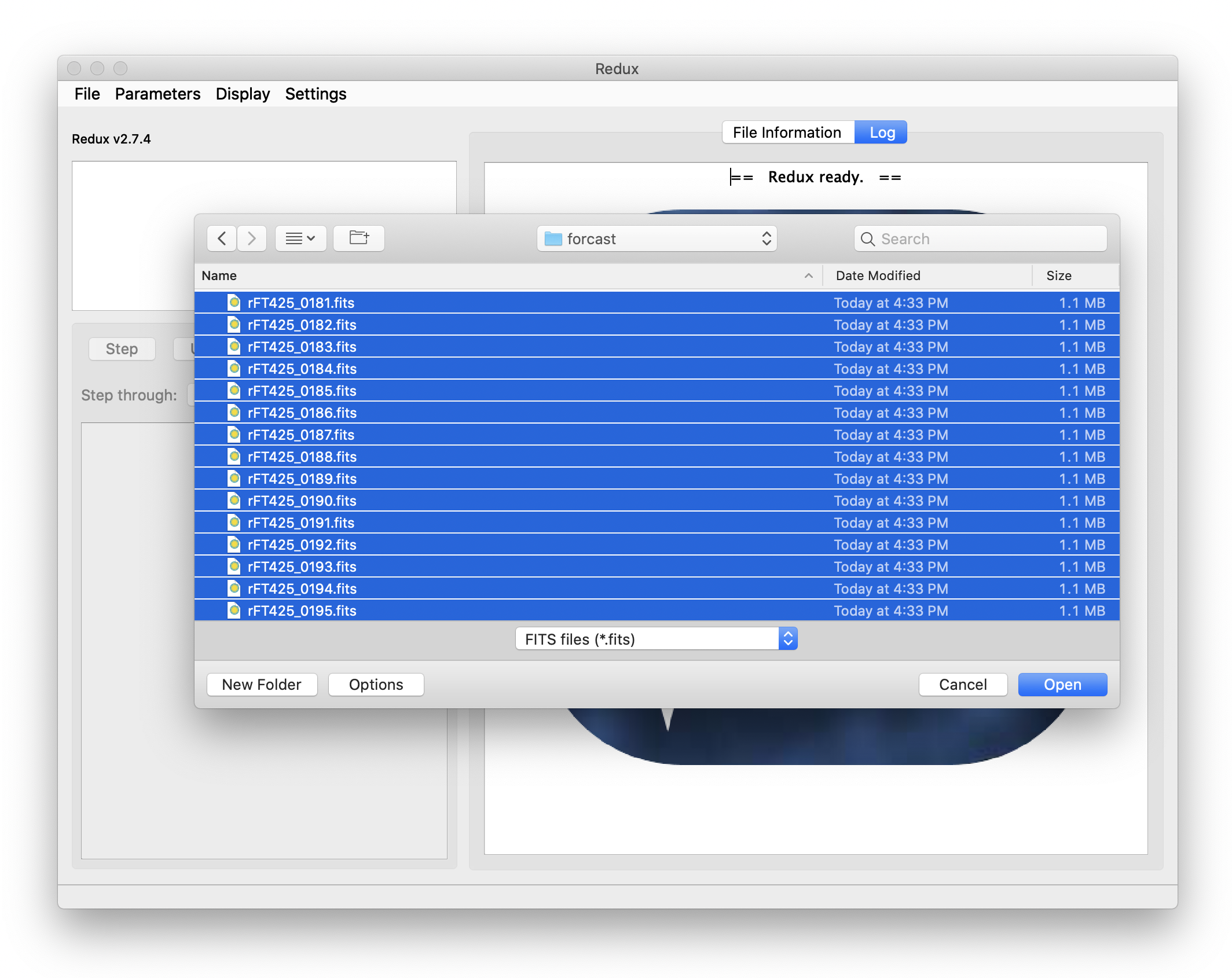
Fig. 125 Open new reduction.¶
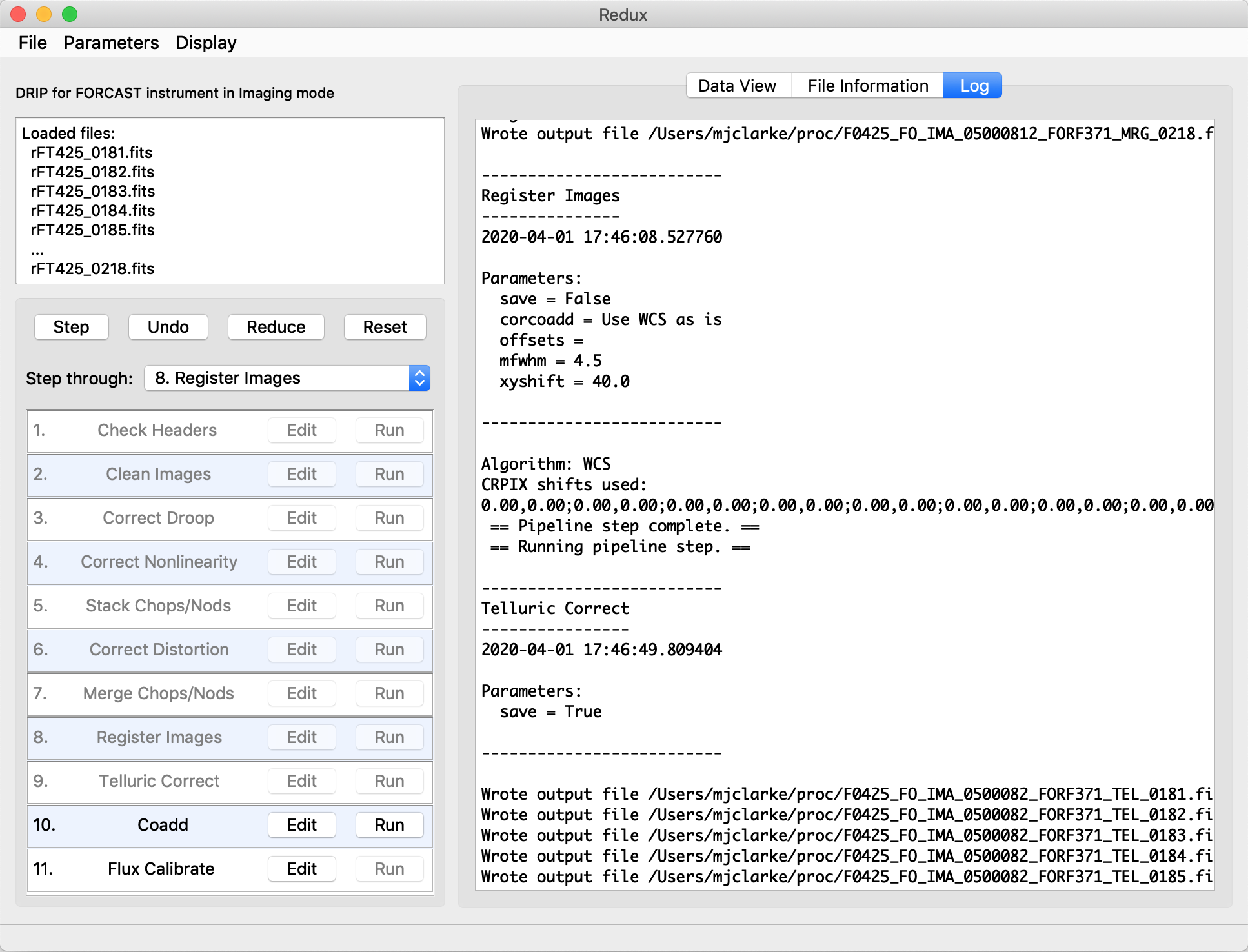
Fig. 126 Sample reduction steps. Log output from the pipeline is displayed in the Log tab.¶
Each reduction step has a number of parameters that can be edited before running the step. To examine or edit these parameters, click the Edit button next to the step name to bring up the parameter editor for that step (Fig. 127). Within the parameter editor, all values may be edited. Click OK to save the edited values and close the window. Click Reset to restore any edited values to their last saved values. Click Restore Defaults to reset all values to their stored defaults. Click Cancel to discard all changes to the parameters and close the editor window.
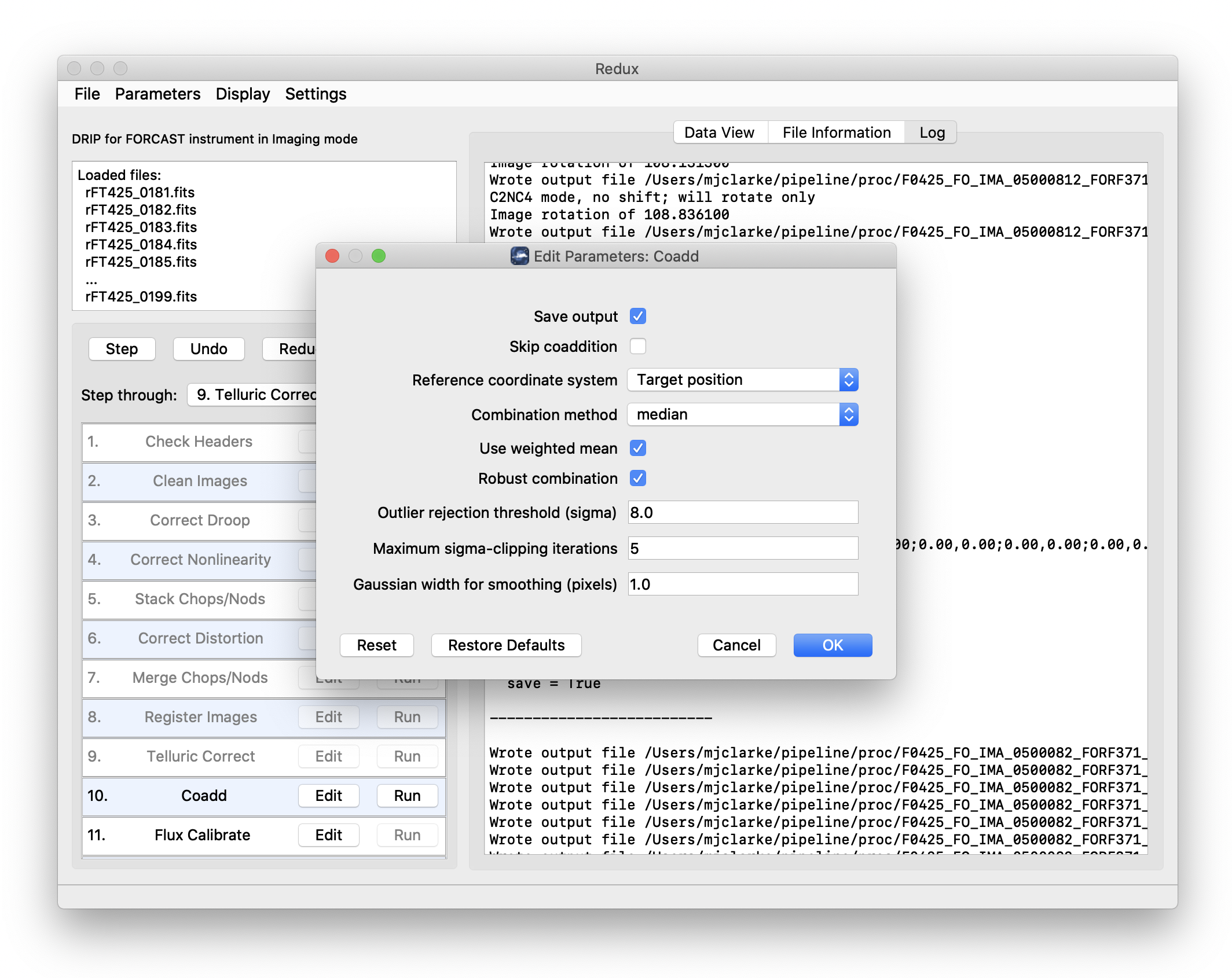
Fig. 127 Sample parameter editor for a pipeline step.¶
The current set of parameters can be displayed, saved to a file, or reset all at once using the Parameters menu. A previously saved set of parameters can also be restored for use with the current reduction (Parameters -> Load Parameters).
After all parameters for a step have been examined and set to the user’s satisfaction, a processing step can be run on all loaded files either by clicking Step, or the Run button next to the step name. Each processing step must be run in order, but if a processing step is selected in the Step through: widget, then clicking Step will treat all steps up through the selected step as a single step and run them all at once. When a step has been completed, its buttons will be grayed out and inaccessible. It is possible to undo one previous step by clicking Undo. All remaining steps can be run at once by clicking Reduce. After each step, the results of the processing may be displayed in a data viewer. After running a pipeline step or reduction, click Reset to restore the reduction to the initial state, without resetting parameter values.
Files can be added to the reduction set (File -> Add Files) or removed from the reduction set (File -> Remove Files), but either action will reset the reduction for all loaded files. Select the File Information tab to display a table of information about the currently loaded files (Fig. 128).
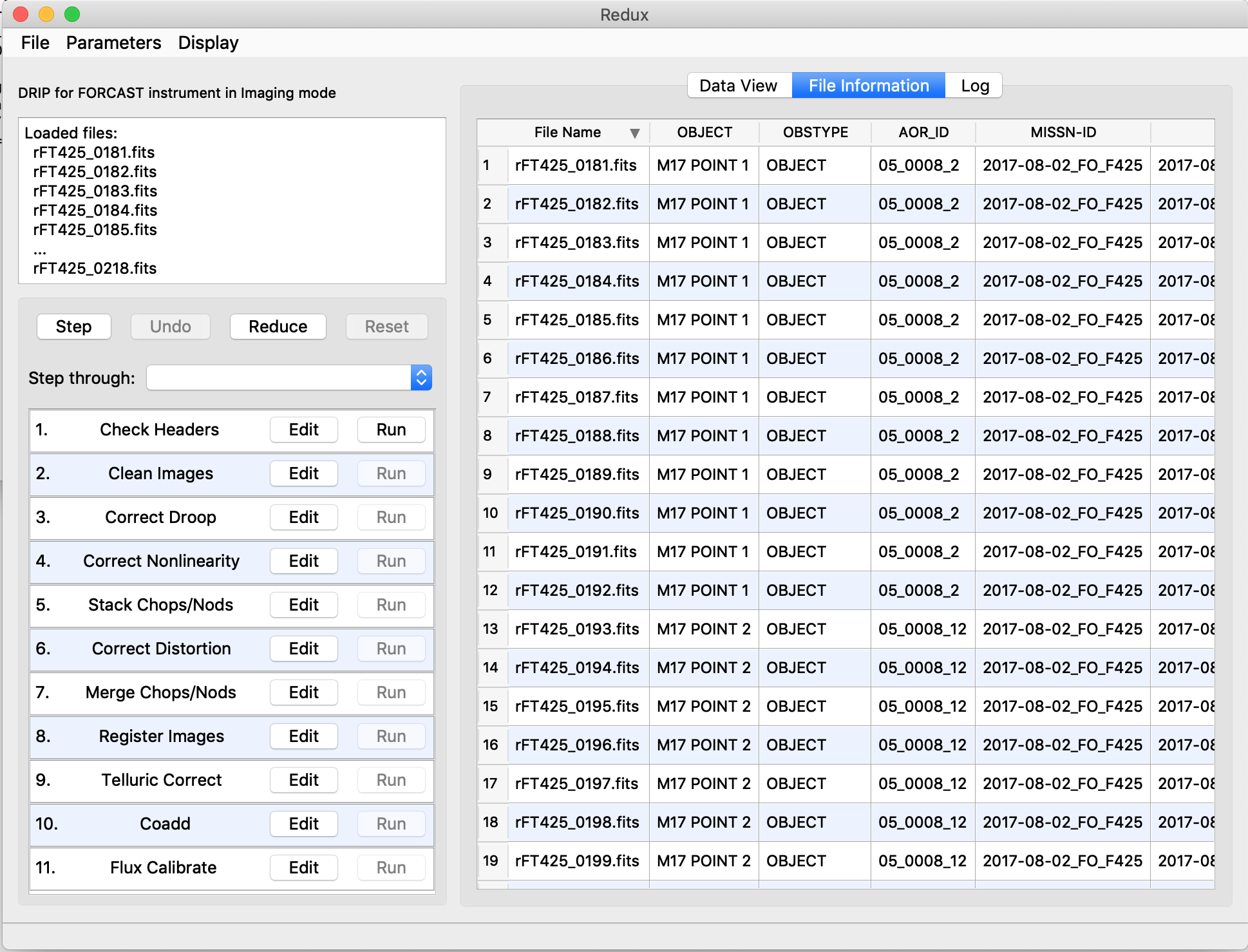
Fig. 128 File information table.¶
Display Features¶
The Redux GUI displays images for quality analysis and display (QAD) in the DS9 FITS viewer. DS9 is a standalone image display tool with an extensive feature set. See the SAO DS9 site (http://ds9.si.edu/) for more usage information.
After each pipeline step completes, Redux may load the produced images into DS9. Some display options may be customized directly in DS9; some commonly used options are accessible from the Redux interface, in the Data View tab (Fig. 129).
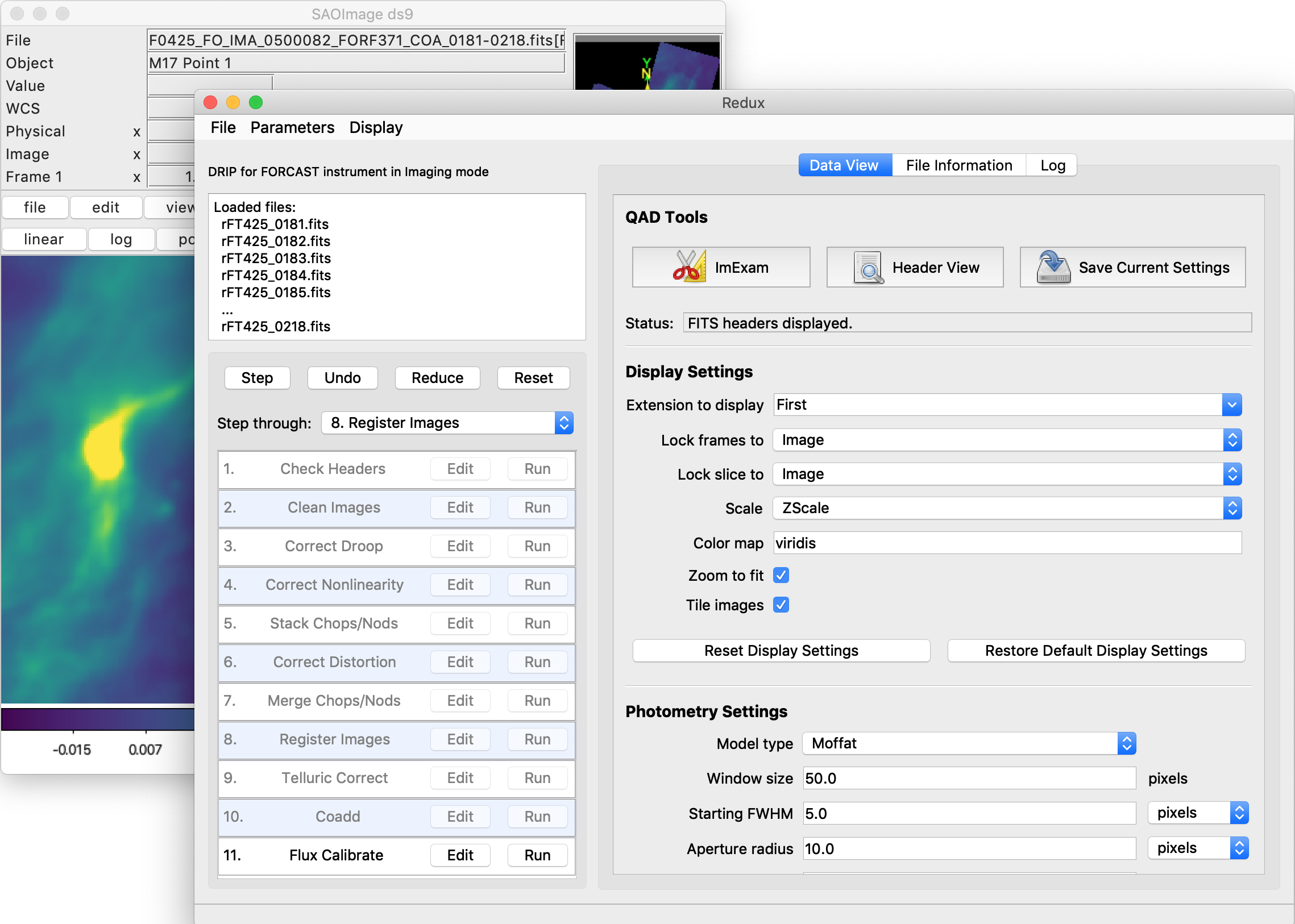
Fig. 129 Data viewer settings and tools.¶
From the Redux interface, the Display Settings can be used to:
Set the FITS extension to display (First, or edit to enter a specific extension), or specify that all extensions should be displayed in a cube or in separate frames.
Lock individual frames together, in image or WCS coordinates.
Lock cube slices for separate frames together, in image or WCS coordinates.
Set the image scaling scheme.
Set a default color map.
Zoom to fit image after loading.
Tile image frames, rather than displaying a single frame at a time.
Changing any of these options in the Data View tab will cause the currently displayed data to be reloaded, with the new options. Clicking Reset Display Settings will revert any edited options to the last saved values. Clicking Restore Default Display Settings will revert all options to their default values.
In the QAD Tools section of the Data View tab, there are several additional tools available.
Clicking the ImExam button (scissors icon) launches an event loop in DS9. After launching it, bring the DS9 window forward, then use the keyboard to perform interactive analysis tasks:
Type ‘a’ over a source in the image to perform photometry at the cursor location.
Type ‘p’ to plot a pixel-to-pixel comparison of all frames at the cursor location.
Type ‘s’ to compute statistics and plot a histogram of the data at the cursor location.
Type ‘c’ to clear any previous photometry results or active plots.
Type ‘h’ to print a help message.
Type ‘q’ to quit the ImExam loop.
The photometry settings (the image window considered, the model fit,
the aperture sizes, etc.) may be customized in the Photometry Settings.
Plot settings (analysis window size, shared plot axes, etc.) may be
customized in the Plot Settings.
After modifying these settings, they will take effect only for new
apertures or plots (use ‘c’ to clear old ones first). As for the display
settings, the reset button will revert to the last saved values
and the restore button will revert to default values.
For the pixel-to-pixel and histogram plots, if the cursor is contained within
a previously defined DS9 region (and the regions
package is installed),
the plot will consider only pixels within the region. Otherwise, a window
around the cursor is used to generate the plot data. Setting the window
to a blank value in the plot settings will use the entire image.
Clicking the Header button (magnifying glass icon) from the QAD Tools section opens a new window that displays headers from currently loaded FITS files in text form (Fig. 130). The extensions displayed depends on the extension setting selected (in Extension to Display). If a particular extension is selected, only that header will be displayed. If all extensions are selected (either for cube or multi-frame display), all extension headers will be displayed. The buttons at the bottom of the window may be used to find or filter the header text, or generate a table of header keywords. For filter or table display, a comma-separated list of keys may be entered in the text box.
Clicking the Save Current Settings button (disk icon) from the QAD Tools section saves all current display and photometry settings for the current user. This allows the user’s settings to persist across new Redux reductions, and to be loaded when Redux next starts up.
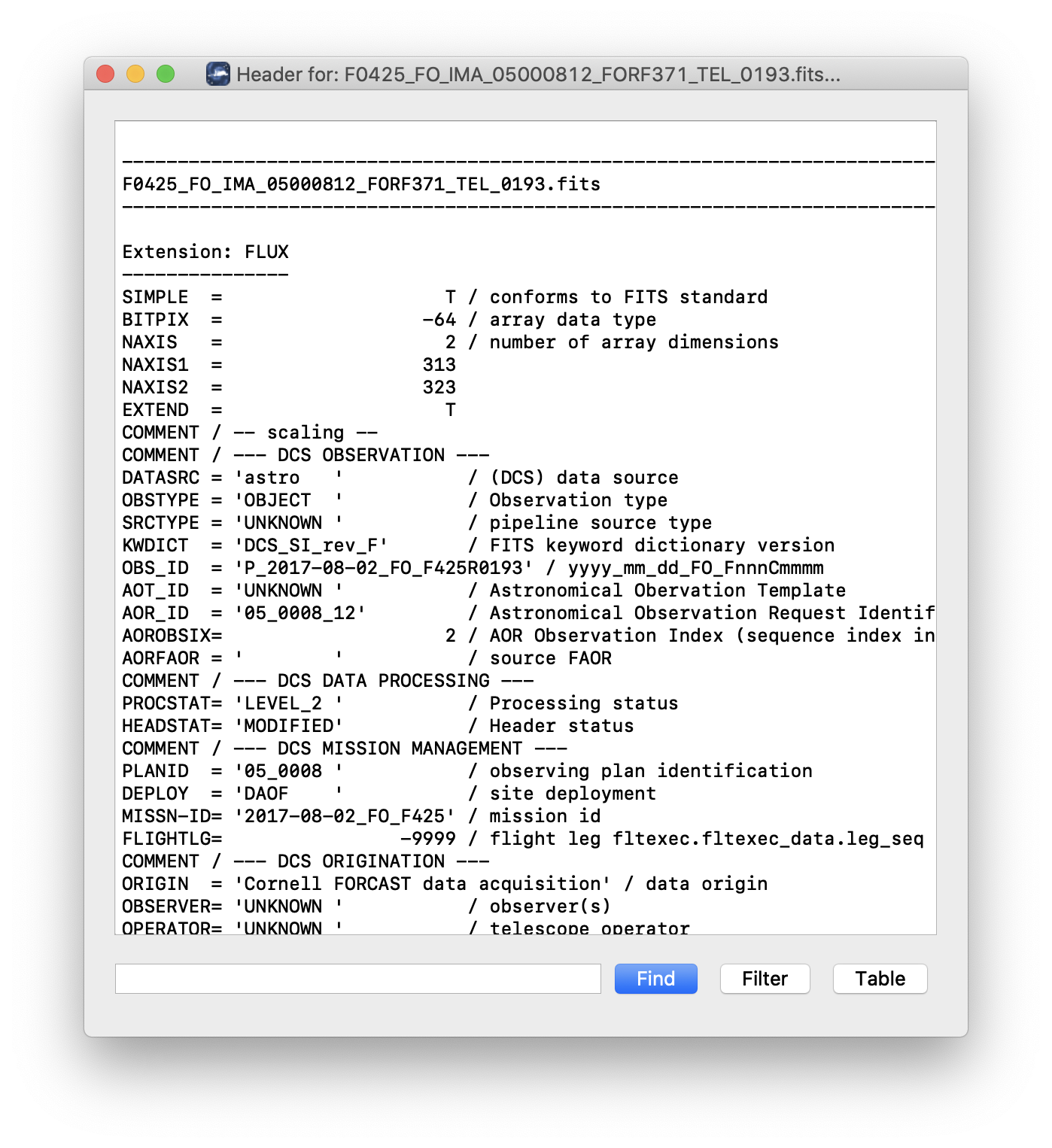
Fig. 130 QAD FITS header viewer.¶
Reference/API¶
Redux Core¶
sofia_redux.pipeline.application Module¶
Run Redux reduction objects from a GUI interface.
Functions¶
|
Run the Redux GUI. |
Classes¶
|
Graphical interface to Redux reduction objects. |
Class Inheritance Diagram¶
digraph inheritance1f8ce58a36 { bgcolor=transparent; rankdir=LR; size=""; "Application" [URL="../../api/sofia_redux.pipeline.application.Application.html#sofia_redux.pipeline.application.Application",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Graphical interface to Redux reduction objects."]; "Interface" -> "Application" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Interface" [URL="../../api/sofia_redux.pipeline.interface.Interface.html#sofia_redux.pipeline.interface.Interface",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Interface to Redux reduction objects."]; }sofia_redux.pipeline.chooser Module¶
Choose Redux reduction objects based on input data.
Classes¶
|
Choose Redux reduction objects. |
Class Inheritance Diagram¶
digraph inheritance513b540efd { bgcolor=transparent; rankdir=LR; size=""; "Chooser" [URL="../../api/sofia_redux.pipeline.chooser.Chooser.html#sofia_redux.pipeline.chooser.Chooser",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Choose Redux reduction objects."]; }sofia_redux.pipeline.configuration Module¶
Redux configuration.
Classes¶
|
Set Redux configuration. |
Class Inheritance Diagram¶
digraph inheritance08c433e7a9 { bgcolor=transparent; rankdir=LR; size=""; "Configuration" [URL="../../api/sofia_redux.pipeline.configuration.Configuration.html#sofia_redux.pipeline.configuration.Configuration",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Set Redux configuration."]; }sofia_redux.pipeline.interface Module¶
Interface to Redux reduction objects.
Classes¶
|
Simple log handler for printing INFO messages. |
|
Interface to Redux reduction objects. |
Class Inheritance Diagram¶
digraph inheritance323ed49b07 { bgcolor=transparent; rankdir=LR; size=""; "Filterer" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",tooltip="A base class for loggers and handlers which allows them to share"]; "Handler" [URL="https://docs.python.org/3/library/logging.html#logging.Handler",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Handler instances dispatch logging events to specific destinations."]; "Filterer" -> "Handler" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Interface" [URL="../../api/sofia_redux.pipeline.interface.Interface.html#sofia_redux.pipeline.interface.Interface",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Interface to Redux reduction objects."]; "StreamHandler" [URL="https://docs.python.org/3/library/logging.handlers.html#logging.StreamHandler",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="A handler class which writes logging records, appropriately formatted,"]; "Handler" -> "StreamHandler" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "TidyLogHandler" [URL="../../api/sofia_redux.pipeline.interface.TidyLogHandler.html#sofia_redux.pipeline.interface.TidyLogHandler",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Simple log handler for printing INFO messages."]; "StreamHandler" -> "TidyLogHandler" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; }sofia_redux.pipeline.parameters Module¶
Base classes for Redux parameter sets.
Classes¶
Ordered dictionary of parameter values for a reduction step. |
|
|
Container class for all parameters needed for a reduction. |
Class Inheritance Diagram¶
digraph inheritance64a4ec79d7 { bgcolor=transparent; rankdir=LR; size=""; "OrderedDict" [URL="https://docs.python.org/3/library/collections.html#collections.OrderedDict",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Dictionary that remembers insertion order"]; "ParameterSet" [URL="../../api/sofia_redux.pipeline.parameters.ParameterSet.html#sofia_redux.pipeline.parameters.ParameterSet",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Ordered dictionary of parameter values for a reduction step."]; "OrderedDict" -> "ParameterSet" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Parameters" [URL="../../api/sofia_redux.pipeline.parameters.Parameters.html#sofia_redux.pipeline.parameters.Parameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Container class for all parameters needed for a reduction."]; }sofia_redux.pipeline.pipe Module¶
Run Redux reduction objects from the command line.
Functions¶
|
Run a pipeline from the command line. |
Classes¶
|
Command-line interface to data reduction steps. |
Class Inheritance Diagram¶
digraph inheritance563b44b32e { bgcolor=transparent; rankdir=LR; size=""; "Interface" [URL="../../api/sofia_redux.pipeline.interface.Interface.html#sofia_redux.pipeline.interface.Interface",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Interface to Redux reduction objects."]; "Pipe" [URL="../../api/sofia_redux.pipeline.pipe.Pipe.html#sofia_redux.pipeline.pipe.Pipe",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Command-line interface to data reduction steps."]; "Interface" -> "Pipe" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; }sofia_redux.pipeline.reduction Module¶
Base class for Redux reduction objects.
Classes¶
Reduction steps and data. |
Class Inheritance Diagram¶
digraph inheritance012fdc6b61 { bgcolor=transparent; rankdir=LR; size=""; "Reduction" [URL="../../api/sofia_redux.pipeline.reduction.Reduction.html#sofia_redux.pipeline.reduction.Reduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction steps and data."]; }sofia_redux.pipeline.viewer Module¶
Base class for Redux viewers.
Classes¶
|
Parent class for Redux data viewers. |
Class Inheritance Diagram¶
digraph inheritance8958b58fe1 { bgcolor=transparent; rankdir=LR; size=""; "Viewer" [URL="../../api/sofia_redux.pipeline.viewer.Viewer.html#sofia_redux.pipeline.viewer.Viewer",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Parent class for Redux data viewers."]; }Redux GUI¶
sofia_redux.pipeline.gui.main Module¶
Main window for Redux Qt5 GUI.
Classes¶
|
Redux Qt5 GUI main window. |
Class Inheritance Diagram¶
digraph inheritance4a3c077d0f { bgcolor=transparent; rankdir=LR; size=""; "QMainWindow" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",tooltip="QMainWindow(parent: Optional[QWidget] = None, flags: Union[Qt.WindowFlags, Qt.WindowType] = Qt.WindowFlags())"]; "QWidget" -> "QMainWindow" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QObject" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",tooltip="QObject(parent: Optional[QObject] = None)"]; "wrapper" -> "QObject" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QPaintDevice" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",tooltip="QPaintDevice()"]; "simplewrapper" -> "QPaintDevice" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QWidget" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",tooltip="QWidget(parent: Optional[QWidget] = None, flags: Union[Qt.WindowFlags, Qt.WindowType] = Qt.WindowFlags())"]; "QObject" -> "QWidget" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QPaintDevice" -> "QWidget" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "ReduxMainWindow" [URL="../../api/sofia_redux.pipeline.gui.main.ReduxMainWindow.html#sofia_redux.pipeline.gui.main.ReduxMainWindow",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Redux Qt5 GUI main window."]; "QMainWindow" -> "ReduxMainWindow" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Ui_MainWindow" -> "ReduxMainWindow" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Ui_MainWindow" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled"]; "simplewrapper" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled"]; "wrapper" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled"]; "simplewrapper" -> "wrapper" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; }sofia_redux.pipeline.gui.matplotlib_viewer Module¶
Basic Matplotlib viewer for image/plot display.
Classes¶
|
Show plot data in a separate window. |
|
Basic Matplotlib viewer. |
Class Inheritance Diagram¶
digraph inheritance9b4cd66824 { bgcolor=transparent; rankdir=LR; size=""; "MatplotlibPlot" [URL="../../api/sofia_redux.pipeline.gui.matplotlib_viewer.MatplotlibPlot.html#sofia_redux.pipeline.gui.matplotlib_viewer.MatplotlibPlot",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Show plot data in a separate window."]; "QDialog" -> "MatplotlibPlot" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "MatplotlibViewer" [URL="../../api/sofia_redux.pipeline.gui.matplotlib_viewer.MatplotlibViewer.html#sofia_redux.pipeline.gui.matplotlib_viewer.MatplotlibViewer",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top"]; "Viewer" -> "MatplotlibViewer" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QDialog" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",tooltip="QDialog(parent: Optional[QWidget] = None, flags: Union[Qt.WindowFlags, Qt.WindowType] = Qt.WindowFlags())"]; "QWidget" -> "QDialog" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QObject" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",tooltip="QObject(parent: Optional[QObject] = None)"]; "wrapper" -> "QObject" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QPaintDevice" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",tooltip="QPaintDevice()"]; "simplewrapper" -> "QPaintDevice" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QWidget" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",tooltip="QWidget(parent: Optional[QWidget] = None, flags: Union[Qt.WindowFlags, Qt.WindowType] = Qt.WindowFlags())"]; "QObject" -> "QWidget" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QPaintDevice" -> "QWidget" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Viewer" [URL="../../api/sofia_redux.pipeline.viewer.Viewer.html#sofia_redux.pipeline.viewer.Viewer",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Parent class for Redux data viewers."]; "simplewrapper" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled"]; "wrapper" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled"]; "simplewrapper" -> "wrapper" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; }sofia_redux.pipeline.gui.qad_viewer Module¶
Redux Viewer using QAD modules for display to DS9.
Classes¶
|
Settings widget for QAD Viewer. |
Redux Viewer interface to DS9 and the Eye of SOFIA. |
Class Inheritance Diagram¶
digraph inheritance1c229e5394 { bgcolor=transparent; rankdir=LR; size=""; "QADViewer" [URL="../../api/sofia_redux.pipeline.gui.qad_viewer.QADViewer.html#sofia_redux.pipeline.gui.qad_viewer.QADViewer",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Redux Viewer interface to DS9 and the Eye of SOFIA."]; "Viewer" -> "QADViewer" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QADViewerSettings" [URL="../../api/sofia_redux.pipeline.gui.qad_viewer.QADViewerSettings.html#sofia_redux.pipeline.gui.qad_viewer.QADViewerSettings",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Settings widget for QAD Viewer."]; "QWidget" -> "QADViewerSettings" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Ui_Form" -> "QADViewerSettings" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QObject" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",tooltip="QObject(parent: Optional[QObject] = None)"]; "wrapper" -> "QObject" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QPaintDevice" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",tooltip="QPaintDevice()"]; "simplewrapper" -> "QPaintDevice" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QWidget" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",tooltip="QWidget(parent: Optional[QWidget] = None, flags: Union[Qt.WindowFlags, Qt.WindowType] = Qt.WindowFlags())"]; "QObject" -> "QWidget" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QPaintDevice" -> "QWidget" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Ui_Form" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled"]; "Viewer" [URL="../../api/sofia_redux.pipeline.viewer.Viewer.html#sofia_redux.pipeline.viewer.Viewer",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Parent class for Redux data viewers."]; "simplewrapper" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled"]; "wrapper" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled"]; "simplewrapper" -> "wrapper" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; }sofia_redux.pipeline.gui.textview Module¶
Text viewer widget for use with QAD and Redux.
Classes¶
|
View, find, and filter text. |
Class Inheritance Diagram¶
digraph inheritancef9c3d4cdfa { bgcolor=transparent; rankdir=LR; size=""; "QDialog" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",tooltip="QDialog(parent: Optional[QWidget] = None, flags: Union[Qt.WindowFlags, Qt.WindowType] = Qt.WindowFlags())"]; "QWidget" -> "QDialog" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QObject" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",tooltip="QObject(parent: Optional[QObject] = None)"]; "wrapper" -> "QObject" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QPaintDevice" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",tooltip="QPaintDevice()"]; "simplewrapper" -> "QPaintDevice" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QWidget" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",tooltip="QWidget(parent: Optional[QWidget] = None, flags: Union[Qt.WindowFlags, Qt.WindowType] = Qt.WindowFlags())"]; "QObject" -> "QWidget" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QPaintDevice" -> "QWidget" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "TextView" [URL="../../api/sofia_redux.pipeline.gui.textview.TextView.html#sofia_redux.pipeline.gui.textview.TextView",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="View, find, and filter text."]; "QDialog" -> "TextView" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Ui_TextWindow" -> "TextView" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Ui_TextWindow" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled"]; "simplewrapper" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled"]; "wrapper" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled"]; "simplewrapper" -> "wrapper" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; }sofia_redux.pipeline.gui.widgets Module¶
Widgets and other useful classes for support of the Redux GUI.
Classes¶
|
Reduction step widget. |
|
Progress bar widget for reduction steps. |
|
Edit parameters for a reduction step. |
|
Model for the File Information table view. |
Custom signals for widgets to emit. |
|
Log handler for a TextEdit-based logging window. |
|
|
Dialog to select files for removal. |
|
View and edit current configuration values. |
|
View and filter current parameter values. |
|
Worker class to run a reduction step. |
|
Worker class to load reduction data. |
|
Worker class to run a general function. |
Class Inheritance Diagram¶
digraph inheritance98e9d5abbe { bgcolor=transparent; rankdir=LR; size=""; "ConfigView" [URL="../../api/sofia_redux.pipeline.gui.widgets.ConfigView.html#sofia_redux.pipeline.gui.widgets.ConfigView",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="View and edit current configuration values."]; "TextView" -> "ConfigView" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "CustomSignals" [URL="../../api/sofia_redux.pipeline.gui.widgets.CustomSignals.html#sofia_redux.pipeline.gui.widgets.CustomSignals",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Custom signals for widgets to emit."]; "QObject" -> "CustomSignals" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "DataTableModel" [URL="../../api/sofia_redux.pipeline.gui.widgets.DataTableModel.html#sofia_redux.pipeline.gui.widgets.DataTableModel",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Model for the File Information table view."]; "QAbstractTableModel" -> "DataTableModel" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "EditParam" [URL="../../api/sofia_redux.pipeline.gui.widgets.EditParam.html#sofia_redux.pipeline.gui.widgets.EditParam",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Edit parameters for a reduction step."]; "QDialog" -> "EditParam" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Ui_Dialog" -> "EditParam" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Filterer" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",tooltip="A base class for loggers and handlers which allows them to share"]; "GeneralRunnable" [URL="../../api/sofia_redux.pipeline.gui.widgets.GeneralRunnable.html#sofia_redux.pipeline.gui.widgets.GeneralRunnable",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Worker class to run a general function."]; "QRunnable" -> "GeneralRunnable" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Handler" [URL="https://docs.python.org/3/library/logging.html#logging.Handler",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Handler instances dispatch logging events to specific destinations."]; "Filterer" -> "Handler" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "LoadRunnable" [URL="../../api/sofia_redux.pipeline.gui.widgets.LoadRunnable.html#sofia_redux.pipeline.gui.widgets.LoadRunnable",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Worker class to load reduction data."]; "QRunnable" -> "LoadRunnable" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "ParamView" [URL="../../api/sofia_redux.pipeline.gui.widgets.ParamView.html#sofia_redux.pipeline.gui.widgets.ParamView",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="View and filter current parameter values."]; "TextView" -> "ParamView" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "PipeStep" [URL="../../api/sofia_redux.pipeline.gui.widgets.PipeStep.html#sofia_redux.pipeline.gui.widgets.PipeStep",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction step widget."]; "QWidget" -> "PipeStep" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Ui_Form" -> "PipeStep" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "ProgressFrame" [URL="../../api/sofia_redux.pipeline.gui.widgets.ProgressFrame.html#sofia_redux.pipeline.gui.widgets.ProgressFrame",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Progress bar widget for reduction steps."]; "QWidget" -> "ProgressFrame" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Ui_ProgressFrame" -> "ProgressFrame" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QAbstractItemModel" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",tooltip="QAbstractItemModel(parent: Optional[QObject] = None)"]; "QObject" -> "QAbstractItemModel" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QAbstractTableModel" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",tooltip="QAbstractTableModel(parent: Optional[QObject] = None)"]; "QAbstractItemModel" -> "QAbstractTableModel" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QDialog" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",tooltip="QDialog(parent: Optional[QWidget] = None, flags: Union[Qt.WindowFlags, Qt.WindowType] = Qt.WindowFlags())"]; "QWidget" -> "QDialog" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QObject" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",tooltip="QObject(parent: Optional[QObject] = None)"]; "wrapper" -> "QObject" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QPaintDevice" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",tooltip="QPaintDevice()"]; "simplewrapper" -> "QPaintDevice" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QRunnable" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",tooltip="QRunnable()"]; "wrapper" -> "QRunnable" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QWidget" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",tooltip="QWidget(parent: Optional[QWidget] = None, flags: Union[Qt.WindowFlags, Qt.WindowType] = Qt.WindowFlags())"]; "QObject" -> "QWidget" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QPaintDevice" -> "QWidget" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "RemoveFilesDialog" [URL="../../api/sofia_redux.pipeline.gui.widgets.RemoveFilesDialog.html#sofia_redux.pipeline.gui.widgets.RemoveFilesDialog",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Dialog to select files for removal."]; "QDialog" -> "RemoveFilesDialog" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Ui_Dialog" -> "RemoveFilesDialog" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "StepRunnable" [URL="../../api/sofia_redux.pipeline.gui.widgets.StepRunnable.html#sofia_redux.pipeline.gui.widgets.StepRunnable",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Worker class to run a reduction step."]; "QRunnable" -> "StepRunnable" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "TextEditLogger" [URL="../../api/sofia_redux.pipeline.gui.widgets.TextEditLogger.html#sofia_redux.pipeline.gui.widgets.TextEditLogger",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Log handler for a TextEdit-based logging window."]; "Handler" -> "TextEditLogger" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "TextView" [URL="../../api/sofia_redux.pipeline.gui.textview.TextView.html#sofia_redux.pipeline.gui.textview.TextView",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="View, find, and filter text."]; "QDialog" -> "TextView" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Ui_TextWindow" -> "TextView" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Ui_Dialog" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled"]; "Ui_Dialog" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled"]; "Ui_Form" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled"]; "Ui_ProgressFrame" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled"]; "Ui_TextWindow" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled"]; "simplewrapper" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled"]; "wrapper" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled"]; "simplewrapper" -> "wrapper" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; }QAD Viewer¶
sofia_redux.pipeline.gui.qad.qad_app Module¶
Standalone front-end for QAD display tool.
Functions¶
|
Quality Analysis and Display tool (QAD). |
sofia_redux.pipeline.gui.qad.qad_dialogs Module¶
Dialogs for the QAD standalone GUI.
Classes¶
|
Retrieve user preferences for display parameters. |
|
Retrieve user preferences for photometry parameters. |
|
Retrieve user preferences for plot parameters. |
Class Inheritance Diagram¶
digraph inheritance2fc94ce8e3 { bgcolor=transparent; rankdir=LR; size=""; "DispSettingsDialog" [URL="../../api/sofia_redux.pipeline.gui.qad.qad_dialogs.DispSettingsDialog.html#sofia_redux.pipeline.gui.qad.qad_dialogs.DispSettingsDialog",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Retrieve user preferences for display parameters."]; "QDialog" -> "DispSettingsDialog" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Ui_DisplayDialog" -> "DispSettingsDialog" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "PhotSettingsDialog" [URL="../../api/sofia_redux.pipeline.gui.qad.qad_dialogs.PhotSettingsDialog.html#sofia_redux.pipeline.gui.qad.qad_dialogs.PhotSettingsDialog",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Retrieve user preferences for photometry parameters."]; "QDialog" -> "PhotSettingsDialog" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Ui_PhotometryDialog" -> "PhotSettingsDialog" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "PlotSettingsDialog" [URL="../../api/sofia_redux.pipeline.gui.qad.qad_dialogs.PlotSettingsDialog.html#sofia_redux.pipeline.gui.qad.qad_dialogs.PlotSettingsDialog",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Retrieve user preferences for plot parameters."]; "QDialog" -> "PlotSettingsDialog" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Ui_PlotSettingsDialog" -> "PlotSettingsDialog" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QDialog" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",tooltip="QDialog(parent: Optional[QWidget] = None, flags: Union[Qt.WindowFlags, Qt.WindowType] = Qt.WindowFlags())"]; "QWidget" -> "QDialog" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QObject" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",tooltip="QObject(parent: Optional[QObject] = None)"]; "wrapper" -> "QObject" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QPaintDevice" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",tooltip="QPaintDevice()"]; "simplewrapper" -> "QPaintDevice" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QWidget" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",tooltip="QWidget(parent: Optional[QWidget] = None, flags: Union[Qt.WindowFlags, Qt.WindowType] = Qt.WindowFlags())"]; "QObject" -> "QWidget" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QPaintDevice" -> "QWidget" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Ui_DisplayDialog" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled"]; "Ui_PhotometryDialog" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled"]; "Ui_PlotSettingsDialog" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled"]; "simplewrapper" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled"]; "wrapper" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled"]; "simplewrapper" -> "wrapper" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; }sofia_redux.pipeline.gui.qad.qad_headview Module¶
FITS header viewing widget.
Classes¶
|
View, find, and filter text from FITS headers. |
Class Inheritance Diagram¶
digraph inheritance16bd560c6d { bgcolor=transparent; rankdir=LR; size=""; "HeaderViewer" [URL="../../api/sofia_redux.pipeline.gui.qad.qad_headview.HeaderViewer.html#sofia_redux.pipeline.gui.qad.qad_headview.HeaderViewer",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="View, find, and filter text from FITS headers."]; "TextView" -> "HeaderViewer" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QDialog" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",tooltip="QDialog(parent: Optional[QWidget] = None, flags: Union[Qt.WindowFlags, Qt.WindowType] = Qt.WindowFlags())"]; "QWidget" -> "QDialog" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QObject" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",tooltip="QObject(parent: Optional[QObject] = None)"]; "wrapper" -> "QObject" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QPaintDevice" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",tooltip="QPaintDevice()"]; "simplewrapper" -> "QPaintDevice" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QWidget" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",tooltip="QWidget(parent: Optional[QWidget] = None, flags: Union[Qt.WindowFlags, Qt.WindowType] = Qt.WindowFlags())"]; "QObject" -> "QWidget" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QPaintDevice" -> "QWidget" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "TextView" [URL="../../api/sofia_redux.pipeline.gui.textview.TextView.html#sofia_redux.pipeline.gui.textview.TextView",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="View, find, and filter text."]; "QDialog" -> "TextView" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Ui_TextWindow" -> "TextView" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Ui_TextWindow" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled"]; "simplewrapper" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled"]; "wrapper" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled"]; "simplewrapper" -> "wrapper" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; }sofia_redux.pipeline.gui.qad.qad_imview Module¶
DS9 Image Viewer for QAD.
Classes¶
View FITS images in DS9. |
Class Inheritance Diagram¶
digraph inheritancea08f45fd16 { bgcolor=transparent; rankdir=LR; size=""; "QADImView" [URL="../../api/sofia_redux.pipeline.gui.qad.qad_imview.QADImView.html#sofia_redux.pipeline.gui.qad.qad_imview.QADImView",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="View FITS images in DS9."]; }sofia_redux.pipeline.gui.qad.qad_main_panel Module¶
Main GUI window for the QAD standalone tool.
Classes¶
QAD Qt5 GUI main window. |
Class Inheritance Diagram¶
digraph inheritance72664c2021 { bgcolor=transparent; rankdir=LR; size=""; "QADMainWindow" [URL="../../api/sofia_redux.pipeline.gui.qad.qad_main_panel.QADMainWindow.html#sofia_redux.pipeline.gui.qad.qad_main_panel.QADMainWindow",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="QAD Qt5 GUI main window."]; "QMainWindow" -> "QADMainWindow" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Ui_MainWindow" -> "QADMainWindow" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QMainWindow" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",tooltip="QMainWindow(parent: Optional[QWidget] = None, flags: Union[Qt.WindowFlags, Qt.WindowType] = Qt.WindowFlags())"]; "QWidget" -> "QMainWindow" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QObject" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",tooltip="QObject(parent: Optional[QObject] = None)"]; "wrapper" -> "QObject" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QPaintDevice" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",tooltip="QPaintDevice()"]; "simplewrapper" -> "QPaintDevice" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QWidget" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",tooltip="QWidget(parent: Optional[QWidget] = None, flags: Union[Qt.WindowFlags, Qt.WindowType] = Qt.WindowFlags())"]; "QObject" -> "QWidget" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "QPaintDevice" -> "QWidget" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Ui_MainWindow" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled"]; "simplewrapper" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled"]; "wrapper" [fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled"]; "simplewrapper" -> "wrapper" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; }SOFIA Redux¶
sofia_redux.pipeline.sofia.sofia_app Module¶
Run SOFIA Redux reduction objects interactively.
Functions¶
|
Run the Redux GUI. |
sofia_redux.pipeline.sofia.sofia_chooser Module¶
Choose SOFIA reduction objects based on input data.
Classes¶
Choose SOFIA Redux reduction objects. |
Class Inheritance Diagram¶
digraph inheritancec7c3de6ae8 { bgcolor=transparent; rankdir=LR; size=""; "Chooser" [URL="../../api/sofia_redux.pipeline.chooser.Chooser.html#sofia_redux.pipeline.chooser.Chooser",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Choose Redux reduction objects."]; "SOFIAChooser" [URL="../../api/sofia_redux.pipeline.sofia.sofia_chooser.SOFIAChooser.html#sofia_redux.pipeline.sofia.sofia_chooser.SOFIAChooser",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Choose SOFIA Redux reduction objects."]; "Chooser" -> "SOFIAChooser" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; }sofia_redux.pipeline.sofia.sofia_configuration Module¶
SOFIA Redux configuration.
Classes¶
|
Set Redux configuration for SOFIA pipelines. |
Class Inheritance Diagram¶
digraph inheritanceaa4e673051 { bgcolor=transparent; rankdir=LR; size=""; "Configuration" [URL="../../api/sofia_redux.pipeline.configuration.Configuration.html#sofia_redux.pipeline.configuration.Configuration",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Set Redux configuration."]; "SOFIAConfiguration" [URL="../../api/sofia_redux.pipeline.sofia.sofia_configuration.SOFIAConfiguration.html#sofia_redux.pipeline.sofia.sofia_configuration.SOFIAConfiguration",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Set Redux configuration for SOFIA pipelines."]; "Configuration" -> "SOFIAConfiguration" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; }sofia_redux.pipeline.sofia.sofia_pipe Module¶
Run SOFIA Redux reduction objects from the command line.
Functions¶
|
Run Redux in batch mode. |
sofia_redux.pipeline.sofia.sofia_utilities Module¶
Functions¶
|
Parse aperture parameters from input string. |
|
Parse background parameters from input string. |
sofia_redux.pipeline.sofia.exes_reduction Module¶
EXES Reduction pipeline steps
Classes¶
EXES reduction steps. |
Class Inheritance Diagram¶
digraph inheritancef1a45938f7 { bgcolor=transparent; rankdir=LR; size=""; "EXESReduction" [URL="../../api/sofia_redux.pipeline.sofia.exes_reduction.EXESReduction.html#sofia_redux.pipeline.sofia.exes_reduction.EXESReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="EXES reduction steps."]; "Reduction" -> "EXESReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Reduction" [URL="../../api/sofia_redux.pipeline.reduction.Reduction.html#sofia_redux.pipeline.reduction.Reduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction steps and data."]; }sofia_redux.pipeline.sofia.fifils_reduction Module¶
FIFI-LS Reduction pipeline steps
Classes¶
FIFI-LS reduction steps. |
Class Inheritance Diagram¶
digraph inheritance5c883e74c7 { bgcolor=transparent; rankdir=LR; size=""; "FIFILSReduction" [URL="../../api/sofia_redux.pipeline.sofia.fifils_reduction.FIFILSReduction.html#sofia_redux.pipeline.sofia.fifils_reduction.FIFILSReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FIFI-LS reduction steps."]; "Reduction" -> "FIFILSReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Reduction" [URL="../../api/sofia_redux.pipeline.reduction.Reduction.html#sofia_redux.pipeline.reduction.Reduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction steps and data."]; }sofia_redux.pipeline.sofia.flitecam_imaging_reduction Module¶
FLITECAM Imaging Reduction pipeline steps
Classes¶
FLITECAM imaging reduction steps. |
Class Inheritance Diagram¶
digraph inheritancecc9b5100b8 { bgcolor=transparent; rankdir=LR; size=""; "FLITECAMImagingReduction" [URL="../../api/sofia_redux.pipeline.sofia.flitecam_imaging_reduction.FLITECAMImagingReduction.html#sofia_redux.pipeline.sofia.flitecam_imaging_reduction.FLITECAMImagingReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FLITECAM imaging reduction steps."]; "FLITECAMReduction" -> "FLITECAMImagingReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTImagingReduction" -> "FLITECAMImagingReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FLITECAMReduction" [URL="../../api/sofia_redux.pipeline.sofia.flitecam_reduction.FLITECAMReduction.html#sofia_redux.pipeline.sofia.flitecam_reduction.FLITECAMReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FLITECAM reduction steps."]; "FORCASTReduction" -> "FLITECAMReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTImagingReduction" [URL="../../api/sofia_redux.pipeline.sofia.forcast_imaging_reduction.FORCASTImagingReduction.html#sofia_redux.pipeline.sofia.forcast_imaging_reduction.FORCASTImagingReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FORCAST imaging reduction steps."]; "FORCASTReduction" -> "FORCASTImagingReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTReduction" [URL="../../api/sofia_redux.pipeline.sofia.forcast_reduction.FORCASTReduction.html#sofia_redux.pipeline.sofia.forcast_reduction.FORCASTReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FORCAST reduction steps."]; "Reduction" -> "FORCASTReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Reduction" [URL="../../api/sofia_redux.pipeline.reduction.Reduction.html#sofia_redux.pipeline.reduction.Reduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction steps and data."]; }sofia_redux.pipeline.sofia.flitecam_reduction Module¶
FLITECAM Reduction pipeline steps
Classes¶
FLITECAM reduction steps. |
Class Inheritance Diagram¶
digraph inheritance5923191f94 { bgcolor=transparent; rankdir=LR; size=""; "FLITECAMReduction" [URL="../../api/sofia_redux.pipeline.sofia.flitecam_reduction.FLITECAMReduction.html#sofia_redux.pipeline.sofia.flitecam_reduction.FLITECAMReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FLITECAM reduction steps."]; "FORCASTReduction" -> "FLITECAMReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTReduction" [URL="../../api/sofia_redux.pipeline.sofia.forcast_reduction.FORCASTReduction.html#sofia_redux.pipeline.sofia.forcast_reduction.FORCASTReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FORCAST reduction steps."]; "Reduction" -> "FORCASTReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Reduction" [URL="../../api/sofia_redux.pipeline.reduction.Reduction.html#sofia_redux.pipeline.reduction.Reduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction steps and data."]; }sofia_redux.pipeline.sofia.flitecam_slitcorr_reduction Module¶
FLITECAM Grism Slit Correction Reduction pipeline steps
Classes¶
FORCAST spesctroscopic slit correction reduction steps. |
Class Inheritance Diagram¶
digraph inheritance2b195e4831 { bgcolor=transparent; rankdir=LR; size=""; "FLITECAMReduction" [URL="../../api/sofia_redux.pipeline.sofia.flitecam_reduction.FLITECAMReduction.html#sofia_redux.pipeline.sofia.flitecam_reduction.FLITECAMReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FLITECAM reduction steps."]; "FORCASTReduction" -> "FLITECAMReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FLITECAMSlitcorrReduction" [URL="../../api/sofia_redux.pipeline.sofia.flitecam_slitcorr_reduction.FLITECAMSlitcorrReduction.html#sofia_redux.pipeline.sofia.flitecam_slitcorr_reduction.FLITECAMSlitcorrReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FORCAST spesctroscopic slit correction reduction steps."]; "FLITECAMSpectroscopyReduction" -> "FLITECAMSlitcorrReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTSlitcorrReduction" -> "FLITECAMSlitcorrReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FLITECAMSpectroscopyReduction" [URL="../../api/sofia_redux.pipeline.sofia.flitecam_spectroscopy_reduction.FLITECAMSpectroscopyReduction.html#sofia_redux.pipeline.sofia.flitecam_spectroscopy_reduction.FLITECAMSpectroscopyReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FLITECAM spectroscopy reduction steps."]; "FLITECAMReduction" -> "FLITECAMSpectroscopyReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTSpectroscopyReduction" -> "FLITECAMSpectroscopyReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTReduction" [URL="../../api/sofia_redux.pipeline.sofia.forcast_reduction.FORCASTReduction.html#sofia_redux.pipeline.sofia.forcast_reduction.FORCASTReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FORCAST reduction steps."]; "Reduction" -> "FORCASTReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTSlitcorrReduction" [URL="../../api/sofia_redux.pipeline.sofia.forcast_slitcorr_reduction.FORCASTSlitcorrReduction.html#sofia_redux.pipeline.sofia.forcast_slitcorr_reduction.FORCASTSlitcorrReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FORCAST spesctroscopic slit correction reduction steps."]; "FORCASTSpectroscopyReduction" -> "FORCASTSlitcorrReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTSpectroscopyReduction" [URL="../../api/sofia_redux.pipeline.sofia.forcast_spectroscopy_reduction.FORCASTSpectroscopyReduction.html#sofia_redux.pipeline.sofia.forcast_spectroscopy_reduction.FORCASTSpectroscopyReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FORCAST spectroscopy reduction steps."]; "FORCASTReduction" -> "FORCASTSpectroscopyReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Reduction" [URL="../../api/sofia_redux.pipeline.reduction.Reduction.html#sofia_redux.pipeline.reduction.Reduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction steps and data."]; }sofia_redux.pipeline.sofia.flitecam_spatcal_reduction Module¶
FLITECAM Grism Spatcal Reduction pipeline steps
Classes¶
FLITECAM spectroscopic spatial calibration reduction steps. |
Class Inheritance Diagram¶
digraph inheritance40bf8eee75 { bgcolor=transparent; rankdir=LR; size=""; "FLITECAMReduction" [URL="../../api/sofia_redux.pipeline.sofia.flitecam_reduction.FLITECAMReduction.html#sofia_redux.pipeline.sofia.flitecam_reduction.FLITECAMReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FLITECAM reduction steps."]; "FORCASTReduction" -> "FLITECAMReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FLITECAMSpatcalReduction" [URL="../../api/sofia_redux.pipeline.sofia.flitecam_spatcal_reduction.FLITECAMSpatcalReduction.html#sofia_redux.pipeline.sofia.flitecam_spatcal_reduction.FLITECAMSpatcalReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FLITECAM spectroscopic spatial calibration reduction steps."]; "FLITECAMSpectroscopyReduction" -> "FLITECAMSpatcalReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTSpatcalReduction" -> "FLITECAMSpatcalReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FLITECAMSpectroscopyReduction" [URL="../../api/sofia_redux.pipeline.sofia.flitecam_spectroscopy_reduction.FLITECAMSpectroscopyReduction.html#sofia_redux.pipeline.sofia.flitecam_spectroscopy_reduction.FLITECAMSpectroscopyReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FLITECAM spectroscopy reduction steps."]; "FLITECAMReduction" -> "FLITECAMSpectroscopyReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTSpectroscopyReduction" -> "FLITECAMSpectroscopyReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTReduction" [URL="../../api/sofia_redux.pipeline.sofia.forcast_reduction.FORCASTReduction.html#sofia_redux.pipeline.sofia.forcast_reduction.FORCASTReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FORCAST reduction steps."]; "Reduction" -> "FORCASTReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTSpatcalReduction" [URL="../../api/sofia_redux.pipeline.sofia.forcast_spatcal_reduction.FORCASTSpatcalReduction.html#sofia_redux.pipeline.sofia.forcast_spatcal_reduction.FORCASTSpatcalReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FORCAST spectroscopic spatial calibration reduction steps."]; "FORCASTWavecalReduction" -> "FORCASTSpatcalReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTSpectroscopyReduction" [URL="../../api/sofia_redux.pipeline.sofia.forcast_spectroscopy_reduction.FORCASTSpectroscopyReduction.html#sofia_redux.pipeline.sofia.forcast_spectroscopy_reduction.FORCASTSpectroscopyReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FORCAST spectroscopy reduction steps."]; "FORCASTReduction" -> "FORCASTSpectroscopyReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTWavecalReduction" [URL="../../api/sofia_redux.pipeline.sofia.forcast_wavecal_reduction.FORCASTWavecalReduction.html#sofia_redux.pipeline.sofia.forcast_wavecal_reduction.FORCASTWavecalReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FORCAST wavelength calibration reduction steps."]; "FORCASTSpectroscopyReduction" -> "FORCASTWavecalReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Reduction" [URL="../../api/sofia_redux.pipeline.reduction.Reduction.html#sofia_redux.pipeline.reduction.Reduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction steps and data."]; }sofia_redux.pipeline.sofia.flitecam_spectroscopy_reduction Module¶
FLITECAM Spectroscopy Reduction pipeline steps
Classes¶
FLITECAM spectroscopy reduction steps. |
Class Inheritance Diagram¶
digraph inheritance3834ac7a1b { bgcolor=transparent; rankdir=LR; size=""; "FLITECAMReduction" [URL="../../api/sofia_redux.pipeline.sofia.flitecam_reduction.FLITECAMReduction.html#sofia_redux.pipeline.sofia.flitecam_reduction.FLITECAMReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FLITECAM reduction steps."]; "FORCASTReduction" -> "FLITECAMReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FLITECAMSpectroscopyReduction" [URL="../../api/sofia_redux.pipeline.sofia.flitecam_spectroscopy_reduction.FLITECAMSpectroscopyReduction.html#sofia_redux.pipeline.sofia.flitecam_spectroscopy_reduction.FLITECAMSpectroscopyReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FLITECAM spectroscopy reduction steps."]; "FLITECAMReduction" -> "FLITECAMSpectroscopyReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTSpectroscopyReduction" -> "FLITECAMSpectroscopyReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTReduction" [URL="../../api/sofia_redux.pipeline.sofia.forcast_reduction.FORCASTReduction.html#sofia_redux.pipeline.sofia.forcast_reduction.FORCASTReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FORCAST reduction steps."]; "Reduction" -> "FORCASTReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTSpectroscopyReduction" [URL="../../api/sofia_redux.pipeline.sofia.forcast_spectroscopy_reduction.FORCASTSpectroscopyReduction.html#sofia_redux.pipeline.sofia.forcast_spectroscopy_reduction.FORCASTSpectroscopyReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FORCAST spectroscopy reduction steps."]; "FORCASTReduction" -> "FORCASTSpectroscopyReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Reduction" [URL="../../api/sofia_redux.pipeline.reduction.Reduction.html#sofia_redux.pipeline.reduction.Reduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction steps and data."]; }sofia_redux.pipeline.sofia.flitecam_wavecal_reduction Module¶
FLITECAM Grism Wavecal Reduction pipeline steps
Classes¶
FLITECAM wavelength calibration reduction steps. |
Class Inheritance Diagram¶
digraph inheritancec92be3add7 { bgcolor=transparent; rankdir=LR; size=""; "FLITECAMReduction" [URL="../../api/sofia_redux.pipeline.sofia.flitecam_reduction.FLITECAMReduction.html#sofia_redux.pipeline.sofia.flitecam_reduction.FLITECAMReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FLITECAM reduction steps."]; "FORCASTReduction" -> "FLITECAMReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FLITECAMSpectroscopyReduction" [URL="../../api/sofia_redux.pipeline.sofia.flitecam_spectroscopy_reduction.FLITECAMSpectroscopyReduction.html#sofia_redux.pipeline.sofia.flitecam_spectroscopy_reduction.FLITECAMSpectroscopyReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FLITECAM spectroscopy reduction steps."]; "FLITECAMReduction" -> "FLITECAMSpectroscopyReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTSpectroscopyReduction" -> "FLITECAMSpectroscopyReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FLITECAMWavecalReduction" [URL="../../api/sofia_redux.pipeline.sofia.flitecam_wavecal_reduction.FLITECAMWavecalReduction.html#sofia_redux.pipeline.sofia.flitecam_wavecal_reduction.FLITECAMWavecalReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FLITECAM wavelength calibration reduction steps."]; "FLITECAMSpectroscopyReduction" -> "FLITECAMWavecalReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTWavecalReduction" -> "FLITECAMWavecalReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTReduction" [URL="../../api/sofia_redux.pipeline.sofia.forcast_reduction.FORCASTReduction.html#sofia_redux.pipeline.sofia.forcast_reduction.FORCASTReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FORCAST reduction steps."]; "Reduction" -> "FORCASTReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTSpectroscopyReduction" [URL="../../api/sofia_redux.pipeline.sofia.forcast_spectroscopy_reduction.FORCASTSpectroscopyReduction.html#sofia_redux.pipeline.sofia.forcast_spectroscopy_reduction.FORCASTSpectroscopyReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FORCAST spectroscopy reduction steps."]; "FORCASTReduction" -> "FORCASTSpectroscopyReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTWavecalReduction" [URL="../../api/sofia_redux.pipeline.sofia.forcast_wavecal_reduction.FORCASTWavecalReduction.html#sofia_redux.pipeline.sofia.forcast_wavecal_reduction.FORCASTWavecalReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FORCAST wavelength calibration reduction steps."]; "FORCASTSpectroscopyReduction" -> "FORCASTWavecalReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Reduction" [URL="../../api/sofia_redux.pipeline.reduction.Reduction.html#sofia_redux.pipeline.reduction.Reduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction steps and data."]; }sofia_redux.pipeline.sofia.forcast_imaging_reduction Module¶
FORCAST Imaging Reduction pipeline steps
Classes¶
FORCAST imaging reduction steps. |
Class Inheritance Diagram¶
digraph inheritancef3f89981d7 { bgcolor=transparent; rankdir=LR; size=""; "FORCASTImagingReduction" [URL="../../api/sofia_redux.pipeline.sofia.forcast_imaging_reduction.FORCASTImagingReduction.html#sofia_redux.pipeline.sofia.forcast_imaging_reduction.FORCASTImagingReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FORCAST imaging reduction steps."]; "FORCASTReduction" -> "FORCASTImagingReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTReduction" [URL="../../api/sofia_redux.pipeline.sofia.forcast_reduction.FORCASTReduction.html#sofia_redux.pipeline.sofia.forcast_reduction.FORCASTReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FORCAST reduction steps."]; "Reduction" -> "FORCASTReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Reduction" [URL="../../api/sofia_redux.pipeline.reduction.Reduction.html#sofia_redux.pipeline.reduction.Reduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction steps and data."]; }sofia_redux.pipeline.sofia.forcast_reduction Module¶
FORCAST Reduction pipeline steps
Classes¶
FORCAST reduction steps. |
Class Inheritance Diagram¶
digraph inheritance0e4b66460d { bgcolor=transparent; rankdir=LR; size=""; "FORCASTReduction" [URL="../../api/sofia_redux.pipeline.sofia.forcast_reduction.FORCASTReduction.html#sofia_redux.pipeline.sofia.forcast_reduction.FORCASTReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FORCAST reduction steps."]; "Reduction" -> "FORCASTReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Reduction" [URL="../../api/sofia_redux.pipeline.reduction.Reduction.html#sofia_redux.pipeline.reduction.Reduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction steps and data."]; }sofia_redux.pipeline.sofia.forcast_slitcorr_reduction Module¶
FORCAST Grism Calibration Reduction pipeline steps
Classes¶
FORCAST spesctroscopic slit correction reduction steps. |
Class Inheritance Diagram¶
digraph inheritancee2ef19e6e0 { bgcolor=transparent; rankdir=LR; size=""; "FORCASTReduction" [URL="../../api/sofia_redux.pipeline.sofia.forcast_reduction.FORCASTReduction.html#sofia_redux.pipeline.sofia.forcast_reduction.FORCASTReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FORCAST reduction steps."]; "Reduction" -> "FORCASTReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTSlitcorrReduction" [URL="../../api/sofia_redux.pipeline.sofia.forcast_slitcorr_reduction.FORCASTSlitcorrReduction.html#sofia_redux.pipeline.sofia.forcast_slitcorr_reduction.FORCASTSlitcorrReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FORCAST spesctroscopic slit correction reduction steps."]; "FORCASTSpectroscopyReduction" -> "FORCASTSlitcorrReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTSpectroscopyReduction" [URL="../../api/sofia_redux.pipeline.sofia.forcast_spectroscopy_reduction.FORCASTSpectroscopyReduction.html#sofia_redux.pipeline.sofia.forcast_spectroscopy_reduction.FORCASTSpectroscopyReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FORCAST spectroscopy reduction steps."]; "FORCASTReduction" -> "FORCASTSpectroscopyReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Reduction" [URL="../../api/sofia_redux.pipeline.reduction.Reduction.html#sofia_redux.pipeline.reduction.Reduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction steps and data."]; }sofia_redux.pipeline.sofia.forcast_spatcal_reduction Module¶
FORCAST Grism Calibration Reduction pipeline steps
Classes¶
FORCAST spectroscopic spatial calibration reduction steps. |
Class Inheritance Diagram¶
digraph inheritance48c3de536a { bgcolor=transparent; rankdir=LR; size=""; "FORCASTReduction" [URL="../../api/sofia_redux.pipeline.sofia.forcast_reduction.FORCASTReduction.html#sofia_redux.pipeline.sofia.forcast_reduction.FORCASTReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FORCAST reduction steps."]; "Reduction" -> "FORCASTReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTSpatcalReduction" [URL="../../api/sofia_redux.pipeline.sofia.forcast_spatcal_reduction.FORCASTSpatcalReduction.html#sofia_redux.pipeline.sofia.forcast_spatcal_reduction.FORCASTSpatcalReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FORCAST spectroscopic spatial calibration reduction steps."]; "FORCASTWavecalReduction" -> "FORCASTSpatcalReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTSpectroscopyReduction" [URL="../../api/sofia_redux.pipeline.sofia.forcast_spectroscopy_reduction.FORCASTSpectroscopyReduction.html#sofia_redux.pipeline.sofia.forcast_spectroscopy_reduction.FORCASTSpectroscopyReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FORCAST spectroscopy reduction steps."]; "FORCASTReduction" -> "FORCASTSpectroscopyReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTWavecalReduction" [URL="../../api/sofia_redux.pipeline.sofia.forcast_wavecal_reduction.FORCASTWavecalReduction.html#sofia_redux.pipeline.sofia.forcast_wavecal_reduction.FORCASTWavecalReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FORCAST wavelength calibration reduction steps."]; "FORCASTSpectroscopyReduction" -> "FORCASTWavecalReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Reduction" [URL="../../api/sofia_redux.pipeline.reduction.Reduction.html#sofia_redux.pipeline.reduction.Reduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction steps and data."]; }sofia_redux.pipeline.sofia.forcast_spectroscopy_reduction Module¶
FORCAST Spectroscopy Reduction pipeline steps
Classes¶
FORCAST spectroscopy reduction steps. |
Class Inheritance Diagram¶
digraph inheritancedceb2db96d { bgcolor=transparent; rankdir=LR; size=""; "FORCASTReduction" [URL="../../api/sofia_redux.pipeline.sofia.forcast_reduction.FORCASTReduction.html#sofia_redux.pipeline.sofia.forcast_reduction.FORCASTReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FORCAST reduction steps."]; "Reduction" -> "FORCASTReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTSpectroscopyReduction" [URL="../../api/sofia_redux.pipeline.sofia.forcast_spectroscopy_reduction.FORCASTSpectroscopyReduction.html#sofia_redux.pipeline.sofia.forcast_spectroscopy_reduction.FORCASTSpectroscopyReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FORCAST spectroscopy reduction steps."]; "FORCASTReduction" -> "FORCASTSpectroscopyReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Reduction" [URL="../../api/sofia_redux.pipeline.reduction.Reduction.html#sofia_redux.pipeline.reduction.Reduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction steps and data."]; }sofia_redux.pipeline.sofia.forcast_wavecal_reduction Module¶
FORCAST Grism Wavecal Reduction pipeline steps
Classes¶
FORCAST wavelength calibration reduction steps. |
Class Inheritance Diagram¶
digraph inheritance6a6ffab315 { bgcolor=transparent; rankdir=LR; size=""; "FORCASTReduction" [URL="../../api/sofia_redux.pipeline.sofia.forcast_reduction.FORCASTReduction.html#sofia_redux.pipeline.sofia.forcast_reduction.FORCASTReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FORCAST reduction steps."]; "Reduction" -> "FORCASTReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTSpectroscopyReduction" [URL="../../api/sofia_redux.pipeline.sofia.forcast_spectroscopy_reduction.FORCASTSpectroscopyReduction.html#sofia_redux.pipeline.sofia.forcast_spectroscopy_reduction.FORCASTSpectroscopyReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FORCAST spectroscopy reduction steps."]; "FORCASTReduction" -> "FORCASTSpectroscopyReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTWavecalReduction" [URL="../../api/sofia_redux.pipeline.sofia.forcast_wavecal_reduction.FORCASTWavecalReduction.html#sofia_redux.pipeline.sofia.forcast_wavecal_reduction.FORCASTWavecalReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="FORCAST wavelength calibration reduction steps."]; "FORCASTSpectroscopyReduction" -> "FORCASTWavecalReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Reduction" [URL="../../api/sofia_redux.pipeline.reduction.Reduction.html#sofia_redux.pipeline.reduction.Reduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction steps and data."]; }sofia_redux.pipeline.sofia.hawc_reduction Module¶
HAWC Reduction pipeline steps
Classes¶
HAWC+ reduction steps. |
Class Inheritance Diagram¶
digraph inheritancef1f9bec31d { bgcolor=transparent; rankdir=LR; size=""; "HAWCReduction" [URL="../../api/sofia_redux.pipeline.sofia.hawc_reduction.HAWCReduction.html#sofia_redux.pipeline.sofia.hawc_reduction.HAWCReduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="HAWC+ reduction steps."]; "Reduction" -> "HAWCReduction" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Reduction" [URL="../../api/sofia_redux.pipeline.reduction.Reduction.html#sofia_redux.pipeline.reduction.Reduction",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction steps and data."]; }sofia_redux.pipeline.sofia.parameters.exes_parameters Module¶
EXES parameter sets.
Classes¶
|
Reduction parameters for the EXES pipeline. |
Class Inheritance Diagram¶
digraph inheritance9d82111032 { bgcolor=transparent; rankdir=LR; size=""; "EXESParameters" [URL="../../api/sofia_redux.pipeline.sofia.parameters.exes_parameters.EXESParameters.html#sofia_redux.pipeline.sofia.parameters.exes_parameters.EXESParameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction parameters for the EXES pipeline."]; "Parameters" -> "EXESParameters" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Parameters" [URL="../../api/sofia_redux.pipeline.parameters.Parameters.html#sofia_redux.pipeline.parameters.Parameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Container class for all parameters needed for a reduction."]; }sofia_redux.pipeline.sofia.parameters.fifils_parameters Module¶
FIFI-LS parameter sets.
Classes¶
|
Reduction parameters for the FIFI-LS pipeline. |
Class Inheritance Diagram¶
digraph inheritance6b934f7675 { bgcolor=transparent; rankdir=LR; size=""; "FIFILSParameters" [URL="../../api/sofia_redux.pipeline.sofia.parameters.fifils_parameters.FIFILSParameters.html#sofia_redux.pipeline.sofia.parameters.fifils_parameters.FIFILSParameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction parameters for the FIFI-LS pipeline."]; "Parameters" -> "FIFILSParameters" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Parameters" [URL="../../api/sofia_redux.pipeline.parameters.Parameters.html#sofia_redux.pipeline.parameters.Parameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Container class for all parameters needed for a reduction."]; }sofia_redux.pipeline.sofia.parameters.flitecam_imaging_parameters Module¶
FLITECAM Imaging parameter sets.
Classes¶
|
Reduction parameters for the FLITECAM Imaging pipeline. |
Class Inheritance Diagram¶
digraph inheritance2a76b802e5 { bgcolor=transparent; rankdir=LR; size=""; "FLITECAMImagingParameters" [URL="../../api/sofia_redux.pipeline.sofia.parameters.flitecam_imaging_parameters.FLITECAMImagingParameters.html#sofia_redux.pipeline.sofia.parameters.flitecam_imaging_parameters.FLITECAMImagingParameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction parameters for the FLITECAM Imaging pipeline."]; "FLITECAMParameters" -> "FLITECAMImagingParameters" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FLITECAMParameters" [URL="../../api/sofia_redux.pipeline.sofia.parameters.flitecam_parameters.FLITECAMParameters.html#sofia_redux.pipeline.sofia.parameters.flitecam_parameters.FLITECAMParameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction parameters for the FLITECAM pipeline."]; "Parameters" -> "FLITECAMParameters" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Parameters" [URL="../../api/sofia_redux.pipeline.parameters.Parameters.html#sofia_redux.pipeline.parameters.Parameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Container class for all parameters needed for a reduction."]; }sofia_redux.pipeline.sofia.parameters.flitecam_parameters Module¶
FLITECAM parameter sets.
Classes¶
|
Reduction parameters for the FLITECAM pipeline. |
Class Inheritance Diagram¶
digraph inheritance489526707b { bgcolor=transparent; rankdir=LR; size=""; "FLITECAMParameters" [URL="../../api/sofia_redux.pipeline.sofia.parameters.flitecam_parameters.FLITECAMParameters.html#sofia_redux.pipeline.sofia.parameters.flitecam_parameters.FLITECAMParameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction parameters for the FLITECAM pipeline."]; "Parameters" -> "FLITECAMParameters" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Parameters" [URL="../../api/sofia_redux.pipeline.parameters.Parameters.html#sofia_redux.pipeline.parameters.Parameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Container class for all parameters needed for a reduction."]; }sofia_redux.pipeline.sofia.parameters.flitecam_slitcorr_parameters Module¶
FLITECAM parameter sets.
Classes¶
|
Reduction parameters for the FLITECAM grism slitcorr pipeline. |
Class Inheritance Diagram¶
digraph inheritance1c1a0b704c { bgcolor=transparent; rankdir=LR; size=""; "FLITECAMParameters" [URL="../../api/sofia_redux.pipeline.sofia.parameters.flitecam_parameters.FLITECAMParameters.html#sofia_redux.pipeline.sofia.parameters.flitecam_parameters.FLITECAMParameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction parameters for the FLITECAM pipeline."]; "Parameters" -> "FLITECAMParameters" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FLITECAMSlitcorrParameters" [URL="../../api/sofia_redux.pipeline.sofia.parameters.flitecam_slitcorr_parameters.FLITECAMSlitcorrParameters.html#sofia_redux.pipeline.sofia.parameters.flitecam_slitcorr_parameters.FLITECAMSlitcorrParameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction parameters for the FLITECAM grism slitcorr pipeline."]; "FLITECAMSpectroscopyParameters" -> "FLITECAMSlitcorrParameters" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FLITECAMSpectroscopyParameters" [URL="../../api/sofia_redux.pipeline.sofia.parameters.flitecam_spectroscopy_parameters.FLITECAMSpectroscopyParameters.html#sofia_redux.pipeline.sofia.parameters.flitecam_spectroscopy_parameters.FLITECAMSpectroscopyParameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction parameters for the FLITECAM spectroscopy pipeline."]; "FLITECAMParameters" -> "FLITECAMSpectroscopyParameters" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Parameters" [URL="../../api/sofia_redux.pipeline.parameters.Parameters.html#sofia_redux.pipeline.parameters.Parameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Container class for all parameters needed for a reduction."]; }sofia_redux.pipeline.sofia.parameters.flitecam_spatcal_parameters Module¶
FLITECAM parameter sets.
Classes¶
|
Reduction parameters for the FLITECAM grism spatcal pipeline. |
Class Inheritance Diagram¶
digraph inheritance5bf1cb4a9d { bgcolor=transparent; rankdir=LR; size=""; "FLITECAMParameters" [URL="../../api/sofia_redux.pipeline.sofia.parameters.flitecam_parameters.FLITECAMParameters.html#sofia_redux.pipeline.sofia.parameters.flitecam_parameters.FLITECAMParameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction parameters for the FLITECAM pipeline."]; "Parameters" -> "FLITECAMParameters" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FLITECAMSpatcalParameters" [URL="../../api/sofia_redux.pipeline.sofia.parameters.flitecam_spatcal_parameters.FLITECAMSpatcalParameters.html#sofia_redux.pipeline.sofia.parameters.flitecam_spatcal_parameters.FLITECAMSpatcalParameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction parameters for the FLITECAM grism spatcal pipeline."]; "FLITECAMWavecalParameters" -> "FLITECAMSpatcalParameters" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FLITECAMSpectroscopyParameters" [URL="../../api/sofia_redux.pipeline.sofia.parameters.flitecam_spectroscopy_parameters.FLITECAMSpectroscopyParameters.html#sofia_redux.pipeline.sofia.parameters.flitecam_spectroscopy_parameters.FLITECAMSpectroscopyParameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction parameters for the FLITECAM spectroscopy pipeline."]; "FLITECAMParameters" -> "FLITECAMSpectroscopyParameters" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FLITECAMWavecalParameters" [URL="../../api/sofia_redux.pipeline.sofia.parameters.flitecam_wavecal_parameters.FLITECAMWavecalParameters.html#sofia_redux.pipeline.sofia.parameters.flitecam_wavecal_parameters.FLITECAMWavecalParameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction parameters for the FLITECAM grism wavecal pipeline."]; "FLITECAMSpectroscopyParameters" -> "FLITECAMWavecalParameters" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Parameters" [URL="../../api/sofia_redux.pipeline.parameters.Parameters.html#sofia_redux.pipeline.parameters.Parameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Container class for all parameters needed for a reduction."]; }sofia_redux.pipeline.sofia.parameters.flitecam_spectroscopy_parameters Module¶
FLITECAM spectroscopy parameter sets.
Classes¶
|
Reduction parameters for the FLITECAM spectroscopy pipeline. |
Class Inheritance Diagram¶
digraph inheritance1deab669b5 { bgcolor=transparent; rankdir=LR; size=""; "FLITECAMParameters" [URL="../../api/sofia_redux.pipeline.sofia.parameters.flitecam_parameters.FLITECAMParameters.html#sofia_redux.pipeline.sofia.parameters.flitecam_parameters.FLITECAMParameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction parameters for the FLITECAM pipeline."]; "Parameters" -> "FLITECAMParameters" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FLITECAMSpectroscopyParameters" [URL="../../api/sofia_redux.pipeline.sofia.parameters.flitecam_spectroscopy_parameters.FLITECAMSpectroscopyParameters.html#sofia_redux.pipeline.sofia.parameters.flitecam_spectroscopy_parameters.FLITECAMSpectroscopyParameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction parameters for the FLITECAM spectroscopy pipeline."]; "FLITECAMParameters" -> "FLITECAMSpectroscopyParameters" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Parameters" [URL="../../api/sofia_redux.pipeline.parameters.Parameters.html#sofia_redux.pipeline.parameters.Parameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Container class for all parameters needed for a reduction."]; }sofia_redux.pipeline.sofia.parameters.flitecam_wavecal_parameters Module¶
FLITECAM parameter sets.
Classes¶
|
Reduction parameters for the FLITECAM grism wavecal pipeline. |
Class Inheritance Diagram¶
digraph inheritancef25f368702 { bgcolor=transparent; rankdir=LR; size=""; "FLITECAMParameters" [URL="../../api/sofia_redux.pipeline.sofia.parameters.flitecam_parameters.FLITECAMParameters.html#sofia_redux.pipeline.sofia.parameters.flitecam_parameters.FLITECAMParameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction parameters for the FLITECAM pipeline."]; "Parameters" -> "FLITECAMParameters" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FLITECAMSpectroscopyParameters" [URL="../../api/sofia_redux.pipeline.sofia.parameters.flitecam_spectroscopy_parameters.FLITECAMSpectroscopyParameters.html#sofia_redux.pipeline.sofia.parameters.flitecam_spectroscopy_parameters.FLITECAMSpectroscopyParameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction parameters for the FLITECAM spectroscopy pipeline."]; "FLITECAMParameters" -> "FLITECAMSpectroscopyParameters" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FLITECAMWavecalParameters" [URL="../../api/sofia_redux.pipeline.sofia.parameters.flitecam_wavecal_parameters.FLITECAMWavecalParameters.html#sofia_redux.pipeline.sofia.parameters.flitecam_wavecal_parameters.FLITECAMWavecalParameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction parameters for the FLITECAM grism wavecal pipeline."]; "FLITECAMSpectroscopyParameters" -> "FLITECAMWavecalParameters" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Parameters" [URL="../../api/sofia_redux.pipeline.parameters.Parameters.html#sofia_redux.pipeline.parameters.Parameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Container class for all parameters needed for a reduction."]; }sofia_redux.pipeline.sofia.parameters.forcast_imaging_parameters Module¶
FORCAST Imaging parameter sets.
Classes¶
|
Reduction parameters for the FORCAST Imaging pipeline. |
Class Inheritance Diagram¶
digraph inheritance7768d568f3 { bgcolor=transparent; rankdir=LR; size=""; "FORCASTImagingParameters" [URL="../../api/sofia_redux.pipeline.sofia.parameters.forcast_imaging_parameters.FORCASTImagingParameters.html#sofia_redux.pipeline.sofia.parameters.forcast_imaging_parameters.FORCASTImagingParameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction parameters for the FORCAST Imaging pipeline."]; "FORCASTParameters" -> "FORCASTImagingParameters" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTParameters" [URL="../../api/sofia_redux.pipeline.sofia.parameters.forcast_parameters.FORCASTParameters.html#sofia_redux.pipeline.sofia.parameters.forcast_parameters.FORCASTParameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction parameters for the FORCAST pipeline."]; "Parameters" -> "FORCASTParameters" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Parameters" [URL="../../api/sofia_redux.pipeline.parameters.Parameters.html#sofia_redux.pipeline.parameters.Parameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Container class for all parameters needed for a reduction."]; }sofia_redux.pipeline.sofia.parameters.forcast_parameters Module¶
FORCAST parameter sets.
Classes¶
|
Reduction parameters for the FORCAST pipeline. |
Class Inheritance Diagram¶
digraph inheritance8a7de8e4e7 { bgcolor=transparent; rankdir=LR; size=""; "FORCASTParameters" [URL="../../api/sofia_redux.pipeline.sofia.parameters.forcast_parameters.FORCASTParameters.html#sofia_redux.pipeline.sofia.parameters.forcast_parameters.FORCASTParameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction parameters for the FORCAST pipeline."]; "Parameters" -> "FORCASTParameters" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Parameters" [URL="../../api/sofia_redux.pipeline.parameters.Parameters.html#sofia_redux.pipeline.parameters.Parameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Container class for all parameters needed for a reduction."]; }sofia_redux.pipeline.sofia.parameters.forcast_slitcorr_parameters Module¶
FORCAST parameter sets.
Classes¶
|
Reduction parameters for the FORCAST grism slitcorr pipeline. |
Class Inheritance Diagram¶
digraph inheritanceeca9d17a9b { bgcolor=transparent; rankdir=LR; size=""; "FORCASTParameters" [URL="../../api/sofia_redux.pipeline.sofia.parameters.forcast_parameters.FORCASTParameters.html#sofia_redux.pipeline.sofia.parameters.forcast_parameters.FORCASTParameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction parameters for the FORCAST pipeline."]; "Parameters" -> "FORCASTParameters" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTSlitcorrParameters" [URL="../../api/sofia_redux.pipeline.sofia.parameters.forcast_slitcorr_parameters.FORCASTSlitcorrParameters.html#sofia_redux.pipeline.sofia.parameters.forcast_slitcorr_parameters.FORCASTSlitcorrParameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction parameters for the FORCAST grism slitcorr pipeline."]; "FORCASTSpectroscopyParameters" -> "FORCASTSlitcorrParameters" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTSpectroscopyParameters" [URL="../../api/sofia_redux.pipeline.sofia.parameters.forcast_spectroscopy_parameters.FORCASTSpectroscopyParameters.html#sofia_redux.pipeline.sofia.parameters.forcast_spectroscopy_parameters.FORCASTSpectroscopyParameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction parameters for the FORCAST spectroscopy pipeline."]; "FORCASTParameters" -> "FORCASTSpectroscopyParameters" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Parameters" [URL="../../api/sofia_redux.pipeline.parameters.Parameters.html#sofia_redux.pipeline.parameters.Parameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Container class for all parameters needed for a reduction."]; }sofia_redux.pipeline.sofia.parameters.forcast_spatcal_parameters Module¶
FORCAST parameter sets.
Classes¶
|
Reduction parameters for the FORCAST grism spatcal pipeline. |
Class Inheritance Diagram¶
digraph inheritanced814260c39 { bgcolor=transparent; rankdir=LR; size=""; "FORCASTParameters" [URL="../../api/sofia_redux.pipeline.sofia.parameters.forcast_parameters.FORCASTParameters.html#sofia_redux.pipeline.sofia.parameters.forcast_parameters.FORCASTParameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction parameters for the FORCAST pipeline."]; "Parameters" -> "FORCASTParameters" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTSpatcalParameters" [URL="../../api/sofia_redux.pipeline.sofia.parameters.forcast_spatcal_parameters.FORCASTSpatcalParameters.html#sofia_redux.pipeline.sofia.parameters.forcast_spatcal_parameters.FORCASTSpatcalParameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction parameters for the FORCAST grism spatcal pipeline."]; "FORCASTWavecalParameters" -> "FORCASTSpatcalParameters" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTSpectroscopyParameters" [URL="../../api/sofia_redux.pipeline.sofia.parameters.forcast_spectroscopy_parameters.FORCASTSpectroscopyParameters.html#sofia_redux.pipeline.sofia.parameters.forcast_spectroscopy_parameters.FORCASTSpectroscopyParameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction parameters for the FORCAST spectroscopy pipeline."]; "FORCASTParameters" -> "FORCASTSpectroscopyParameters" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTWavecalParameters" [URL="../../api/sofia_redux.pipeline.sofia.parameters.forcast_wavecal_parameters.FORCASTWavecalParameters.html#sofia_redux.pipeline.sofia.parameters.forcast_wavecal_parameters.FORCASTWavecalParameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction parameters for the FORCAST grism wavecal pipeline."]; "FORCASTSpectroscopyParameters" -> "FORCASTWavecalParameters" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Parameters" [URL="../../api/sofia_redux.pipeline.parameters.Parameters.html#sofia_redux.pipeline.parameters.Parameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Container class for all parameters needed for a reduction."]; }sofia_redux.pipeline.sofia.parameters.forcast_spectroscopy_parameters Module¶
FORCAST parameter sets.
Classes¶
|
Reduction parameters for the FORCAST spectroscopy pipeline. |
Class Inheritance Diagram¶
digraph inheritance8ed18cdbea { bgcolor=transparent; rankdir=LR; size=""; "FORCASTParameters" [URL="../../api/sofia_redux.pipeline.sofia.parameters.forcast_parameters.FORCASTParameters.html#sofia_redux.pipeline.sofia.parameters.forcast_parameters.FORCASTParameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction parameters for the FORCAST pipeline."]; "Parameters" -> "FORCASTParameters" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTSpectroscopyParameters" [URL="../../api/sofia_redux.pipeline.sofia.parameters.forcast_spectroscopy_parameters.FORCASTSpectroscopyParameters.html#sofia_redux.pipeline.sofia.parameters.forcast_spectroscopy_parameters.FORCASTSpectroscopyParameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction parameters for the FORCAST spectroscopy pipeline."]; "FORCASTParameters" -> "FORCASTSpectroscopyParameters" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Parameters" [URL="../../api/sofia_redux.pipeline.parameters.Parameters.html#sofia_redux.pipeline.parameters.Parameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Container class for all parameters needed for a reduction."]; }sofia_redux.pipeline.sofia.parameters.forcast_wavecal_parameters Module¶
FORCAST parameter sets.
Classes¶
|
Reduction parameters for the FORCAST grism wavecal pipeline. |
Class Inheritance Diagram¶
digraph inheritance585b458854 { bgcolor=transparent; rankdir=LR; size=""; "FORCASTParameters" [URL="../../api/sofia_redux.pipeline.sofia.parameters.forcast_parameters.FORCASTParameters.html#sofia_redux.pipeline.sofia.parameters.forcast_parameters.FORCASTParameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction parameters for the FORCAST pipeline."]; "Parameters" -> "FORCASTParameters" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTSpectroscopyParameters" [URL="../../api/sofia_redux.pipeline.sofia.parameters.forcast_spectroscopy_parameters.FORCASTSpectroscopyParameters.html#sofia_redux.pipeline.sofia.parameters.forcast_spectroscopy_parameters.FORCASTSpectroscopyParameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction parameters for the FORCAST spectroscopy pipeline."]; "FORCASTParameters" -> "FORCASTSpectroscopyParameters" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "FORCASTWavecalParameters" [URL="../../api/sofia_redux.pipeline.sofia.parameters.forcast_wavecal_parameters.FORCASTWavecalParameters.html#sofia_redux.pipeline.sofia.parameters.forcast_wavecal_parameters.FORCASTWavecalParameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Reduction parameters for the FORCAST grism wavecal pipeline."]; "FORCASTSpectroscopyParameters" -> "FORCASTWavecalParameters" [arrowsize=1.2,arrowtail=empty,dir=back,style="setlinewidth(0.5)"]; "Parameters" [URL="../../api/sofia_redux.pipeline.parameters.Parameters.html#sofia_redux.pipeline.parameters.Parameters",fillcolor=white,fontname="Vera Sans, DejaVu Sans, Liberation Sans, Arial, Helvetica, sans",fontsize=10,height=0.25,margin=0.25,shape=box,style="setlinewidth(0.5),filled",target="_top",tooltip="Container class for all parameters needed for a reduction."]; }sofia_redux.pipeline.sofia.parameters.hawc_parameters Module¶
HAWC parameter sets.
Classes¶
|
Reduction parameters for the HAWC pipeline. |